Constant Arrays in PHP
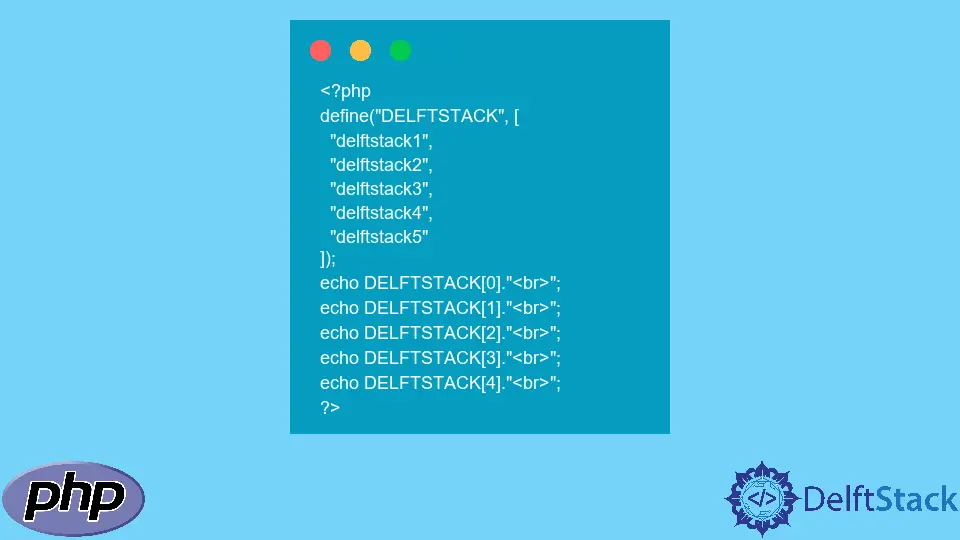
This tutorial demonstrates constant arrays in PHP.
PHP Constant Arrays
The constants are the variables in PHP which are defined once and then never changed and are undefined. The Constant
keyword is the identifier for any value which cannot be changed in PHP.
The name for valid constant variables will always start with a letter or underscore, and it doesn’t include the $
sign like common variables. The constants are always global in scope and can be used across the script.
The array with a constant
keyword is the constant array that cannot be changed or undefined. To create a constant in PHP, we use the define()
keyword where the syntax is:
define(name, value, case-insensitive)
The name
is the constant’s name, the value
is the value for the constant, and the case-insensitive
is used for whether the constant’s name should be insensitive. The default value for case-insensitive
is false
.
Let’s see how to define a simple constant variable in PHP.
<?php
define("DELFTSTACK", "Hello! This is delftstack.com, the best site for tutorials.");
echo DELFTSTACK;
?>
The code above creates a simple constant variable, DELFTSTACK
, with the given value. See output:
Hello! This is delftstack.com, the best site for tutorials.
From PHP 7, the constant arrays are also supported in PHP. We can use the same define()
method to create a constant array in PHP.
See the example.
<?php
define("DELFTSTACK", [
"delftstack1",
"delftstack2",
"delftstack3",
"delftstack4",
"delftstack5"
]);
echo DELFTSTACK[0]."<br>";
echo DELFTSTACK[1]."<br>";
echo DELFTSTACK[2]."<br>";
echo DELFTSTACK[3]."<br>";
echo DELFTSTACK[4]."<br>";
?>
The code above creates a constant array and prints each member of the constant array. See output:
delftstack1
delftstack2
delftstack3
delftstack4
delftstack5
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook