How to Check if File Exists in PHP
Minahil Noor
Feb 02, 2024
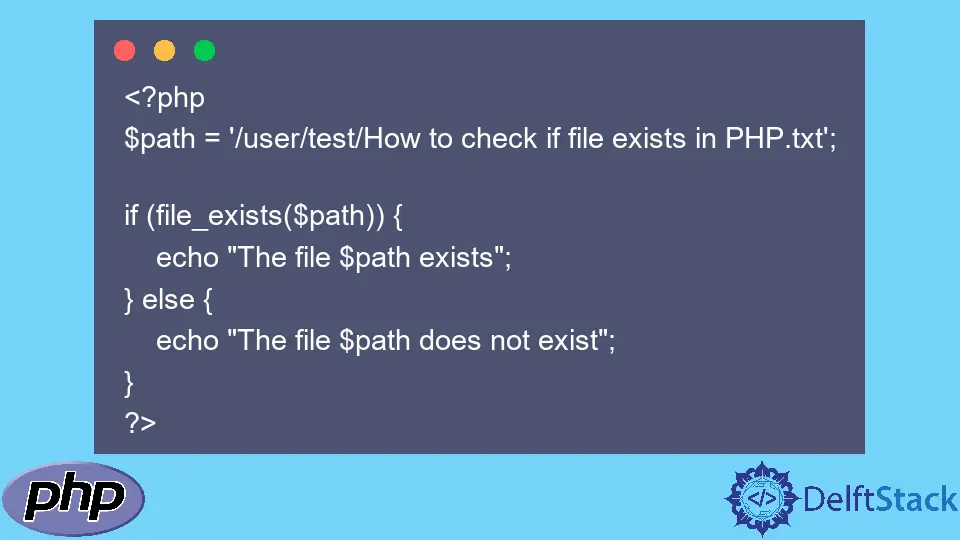
This article will introduce different methods to check if a file exists in PHP.
Use the file_exists()
Function to Check if File Exists in PHP
We have a built-in function in PHP to check if a file exists or not. The function is file_exists()
. This function accepts the file path or name as the parameter and checks if the given file exists or not. The correct syntax to use this function is as follows.
file_exists($path);
This function accepts only one parameter. The detail of its parameter is as follows.
Variables | Description |
---|---|
$path |
It is the path to the file that we want to check. |
The function returns True
if the file exists in the specified directory. The program below shows the way by which we can use the PHP file_exists()
function to check if a file exists.
<?php
$path = '/user/test/How to check if file exists in PHP.txt';
if (file_exists($path)) {
echo "The file $path exists";
} else {
echo "The file $path does not exist";
}
?>
Output:
The file /user/test/How to check if file exists in PHP.txt exists.