How to Call JavaScript Function in PHP
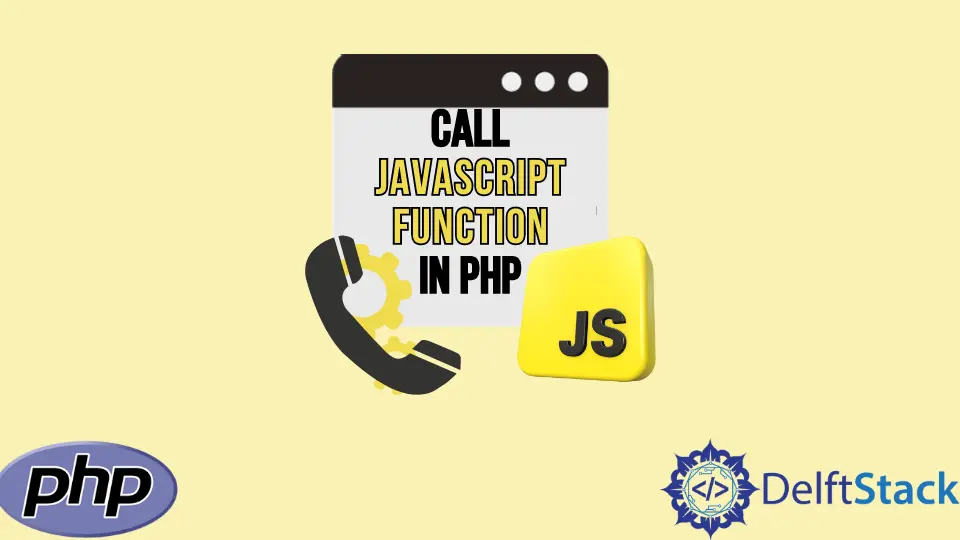
In this article, we will introduce a method to call JavaScript function from PHP. We actually cannot call JavaScript function using PHP as these two languages do not understand each other. The only possible option is outputting the JavaScript function call in PHP. However, we also can write JavaScript in a PHP file and call the function.
Use the echo
Function to Display the JavaScript Function Call in PHP
JavaScript is a client-side scripting language, while PHP is a server-side language. Technically, we cannot invoke JavaScript functions from PHP. But we can display the JavaScript using the echo
function. This method includes the JavaScript function call in the output rather than the JavaScript function in PHP. We will define a JavaScript function first and then call the function. We can wrap the JavaScript invocation of the function with the echo
function in PHP. Here, most work will be done by JavaScript, which is the function declaration and invocation. We will use PHP only to display the function invocation.
For example, create a PHP file index.php
and inside the script
tag write a JavaScript function showMessage()
. Write the alert()
function inside the showMessage()
function. Write the text click here
in the alert()
function. Then, somewhere in the code, open the PHP tag and write the script
tag and call the showMessage()
function inside the tag. Then, wrap the script
tag with the echo
function.
We can also separate each element in the invocation by a single quote. The example is shown below.
echo '<script>', 'showMessage();', '</script>';
When we run the script, then an alert message will pop up saying click here
. We treated the JavaScript function as a string and used it to display with the echo
function. It should be noted that the function should be declared before the invocation. Thus, we called the JavaScript function in PHP using the echo
function.
Example Code:
function showMessage() {
alert('click here');
}
echo "<script> showMessage(); </script>";
Output:
click here
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn