How to Call Function From String in PHP
-
Use
call_user_func()
Call a Function From a String in PHP - Use the Variable Method to Call a Function From a String Stored in a Variable in PHP
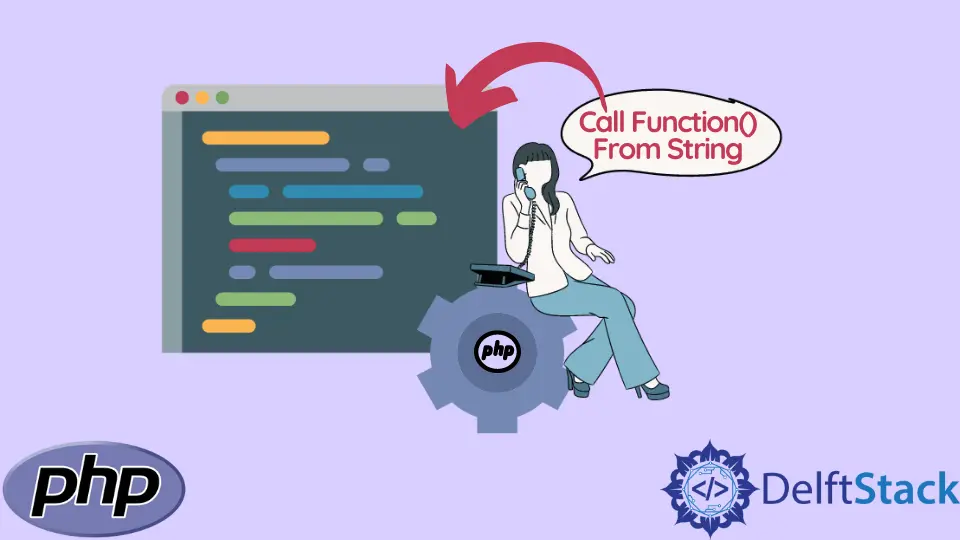
PHP has a built-in function like call_user_func()
to call a function from a string or store it in a variable for the older versions. PHP can also store a function into a variable and use it wherever required.
This tutorial demonstrates different methods to call a function from a string stored in a variable.
Use call_user_func()
Call a Function From a String in PHP
PHP built-in function call_user_func()
can be used for the purpose.
Example:
<?php
function demo_func($name) {
echo "Hello This is Delftstack employee ".$name;
}
$demo_array = array ("John", "Shawn", "Michelle", "Tina");
foreach ($demo_array as $name) {
call_user_func('demo_func', $name);
echo "<br>";
}
?>
The code above calls the function demo_func
with the parameter name.
Output:
Hello This is Delftstack employee John
Hello This is Delftstack employee Shawn
Hello This is Delftstack employee Michelle
Hello This is Delftstack employee Tina
Use the Variable Method to Call a Function From a String Stored in a Variable in PHP
In PHP, we can also store the functions in variables. The function name should be assigned to a variable as a string, and then we can call the function a variable.
Example:
<?php
function demo_func1($name) {
echo "Hello This is Delftstack employee ".$name;
}
$demo_function = 'demo_func1';
$demo_array = array ("John", "Shawn", "Michelle", "Tina");
foreach ($demo_array as $name) {
$demo_function($name);
echo "<br>";
}
?>
The output for this will also be similar to our first example above.
Hello This is Delftstack employee John
Hello This is Delftstack employee Shawn
Hello This is Delftstack employee Michelle
Hello This is Delftstack employee Tina
call_user_func
is the old way to do it. We can directly store the function into variables as a string and call the function by calling the variable.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook