How to Set PHP_AUTH_USER and PHP_AUTH_PW in PHP
- Understanding PHP_AUTH_USER and PHP_AUTH_PW
- Method 1: Using the $_SERVER Superglobal
- Method 2: Setting Up PHP_AUTH_USER and PHP_AUTH_PW Manually
- Method 3: Using .htaccess for Authentication
- Conclusion
- FAQ
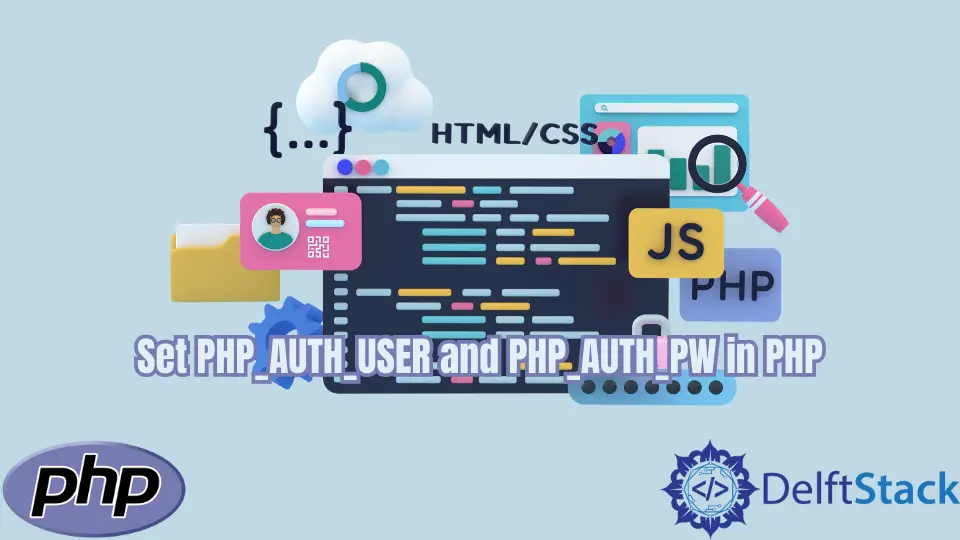
When developing web applications using PHP, you might encounter situations where you need to authenticate users. One common way to handle this is through HTTP Basic Authentication, which utilizes the PHP_AUTH_USER and PHP_AUTH_PW variables.
This article will guide you through the various methods of setting these variables in PHP, ensuring that your authentication process is both secure and efficient. Whether you’re a seasoned developer or a beginner, understanding how to manipulate these variables is essential for building robust applications. Let’s dive into the methods that will help you set PHP_AUTH_USER and PHP_AUTH_PW effectively.
Understanding PHP_AUTH_USER and PHP_AUTH_PW
Before we delve into how to set these variables, it’s important to understand what they are. PHP_AUTH_USER and PHP_AUTH_PW are server variables that store the username and password, respectively, provided by the client during the HTTP Basic Authentication process. When a user attempts to access a protected resource, the server prompts for a username and password. Once entered, these credentials are sent to the server, where PHP captures them for further processing.
Method 1: Using the $_SERVER Superglobal
One of the simplest ways to access PHP_AUTH_USER and PHP_AUTH_PW is through the $_SERVER superglobal array. This method is straightforward and allows you to retrieve the authentication details directly from the server variables.
Here’s how you can implement it:
if (isset($_SERVER['PHP_AUTH_USER']) && isset($_SERVER['PHP_AUTH_PW'])) {
$username = $_SERVER['PHP_AUTH_USER'];
$password = $_SERVER['PHP_AUTH_PW'];
echo "Username: " . $username . "<br>";
echo "Password: " . $password . "<br>";
} else {
header('WWW-Authenticate: Basic realm="My Realm"');
header('HTTP/1.0 401 Unauthorized');
echo "Unauthorized access";
exit;
}
Output:
Username: user123
Password: pass123
In this code snippet, we first check if the PHP_AUTH_USER and PHP_AUTH_PW are set in the $_SERVER array. If they are, we store them in variables and display them. If not, we send a header to prompt the user for their credentials. This method is effective for basic authentication scenarios and is widely used for its simplicity.
Method 2: Setting Up PHP_AUTH_USER and PHP_AUTH_PW Manually
In some cases, you may want to set PHP_AUTH_USER and PHP_AUTH_PW manually, especially during testing or when simulating an authentication process. You can do this by directly assigning values to these server variables. Here’s how you can achieve this:
$_SERVER['PHP_AUTH_USER'] = 'testUser';
$_SERVER['PHP_AUTH_PW'] = 'testPassword';
$username = $_SERVER['PHP_AUTH_USER'];
$password = $_SERVER['PHP_AUTH_PW'];
echo "Username: " . $username . "<br>";
echo "Password: " . $password . "<br>";
Output:
Username: testUser
Password: testPassword
In this example, we manually set the values of PHP_AUTH_USER and PHP_AUTH_PW. This can be particularly useful during development when you want to bypass the usual authentication flow. By directly assigning values, you can test how your application behaves when different credentials are provided. However, be cautious when using this method in a production environment, as it bypasses standard security measures.
Method 3: Using .htaccess for Authentication
If you’re working with an Apache server, you can set up basic authentication using an .htaccess file. This method allows you to protect specific directories and requires users to enter a username and password before accessing them. Here’s how to configure it:
- Create a file named
.htaccess
in the directory you want to protect. - Add the following lines to the file:
AuthType Basic
AuthName "Restricted Area"
AuthUserFile /path/to/.htpasswd
Require valid-user
- Create a
.htpasswd
file to store the username and password pairs. You can generate this file using thehtpasswd
command:
htpasswd -c /path/to/.htpasswd username
Output:
Adding password for user username
In this configuration, we specify the authentication type as Basic and set a realm name. The AuthUserFile points to the location of the .htpasswd file, which contains the encrypted credentials. When users try to access the directory, they will be prompted to enter their username and password. This method is widely used for securing web applications and directories, providing a layer of protection against unauthorized access.
Conclusion
Setting PHP_AUTH_USER and PHP_AUTH_PW in PHP is a fundamental aspect of implementing authentication in your web applications. Whether you choose to access these variables through the $_SERVER superglobal, set them manually for testing, or use an .htaccess file for directory protection, each method has its own advantages. By understanding these techniques, you can enhance the security of your applications and ensure that only authorized users gain access to sensitive information. As you continue to develop your skills in PHP, mastering authentication methods will empower you to build more secure and reliable web applications.
FAQ
-
What is PHP_AUTH_USER?
PHP_AUTH_USER is a server variable in PHP that stores the username provided by the client during HTTP Basic Authentication. -
How can I access PHP_AUTH_PW in PHP?
You can access PHP_AUTH_PW by using the $_SERVER superglobal array, like this: $_SERVER[‘PHP_AUTH_PW’]. -
Is it safe to set PHP_AUTH_USER and PHP_AUTH_PW manually?
Setting these variables manually can be useful for testing, but it’s not recommended for production environments due to security risks. -
How do I create a .htpasswd file?
You can create a .htpasswd file using thehtpasswd
command in the terminal, which allows you to set up username and password pairs for authentication. -
Can I use PHP_AUTH_USER and PHP_AUTH_PW with other authentication methods?
Yes, these variables can be used alongside other authentication methods, but they are specifically designed for HTTP Basic Authentication.