How to Alert Message Using PHP
- Pop-Up Alert Message Taking Value From PHP Variable and JavaScript
- Pop-Up Alert Message Using PHP Function JavaScript
- Pop-Up Alert Message Using an Array or an Object
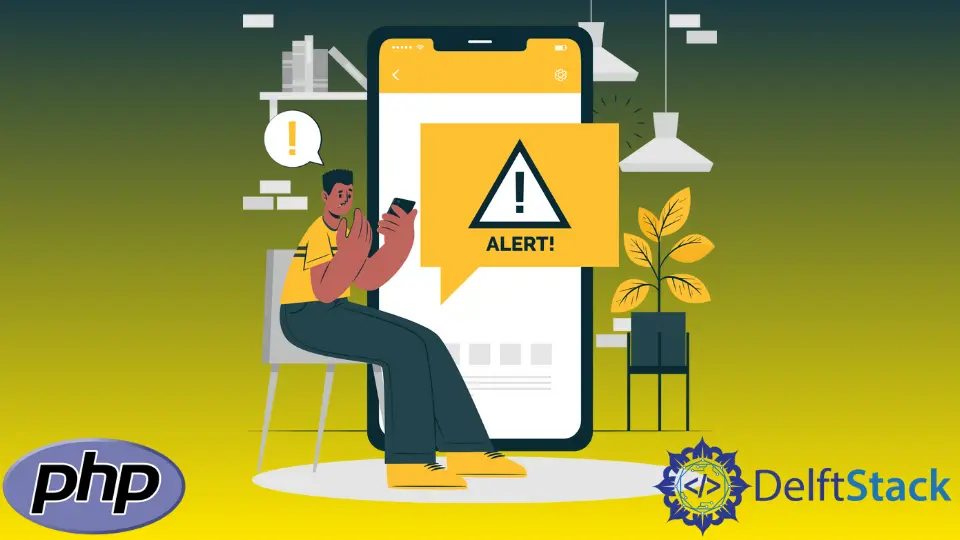
PHP does not have any built-in function for popping alert messages, but we can pop alert messages in PHP using JavaScript. The alert messages are shown in pop-up boxes in the browser, usually used for popping warning messages.
JavaScript helps PHP to show dynamic alert messages in the pop-up box. This tutorial demonstrates how we can use PHP and JavaScript to pop-up alert messages.
Pop-Up Alert Message Taking Value From PHP Variable and JavaScript
To pop-up alert messages in PHP is to put the variable into the javascript alert()
method.
Example:
<?php
// Sending Alert message using PHP variable.
$alert = "This is DEMO WARNING";
echo "<script type='text/javascript'>alert('$alert');</script>";
?>
The above code will pop up an alert box in the browser showing the value of the $alert
variable.
Output:
Pop-Up Alert Message Using PHP Function JavaScript
We can create a PHP function for popping the alert messages.
Example:
<?php
// Sending alert messages using PHP function
$message1= "This is the PHP function alert 1";
$message2= "This is the PHP function alert 2";
function alert($message) {
echo "<script type='text/javascript'>alert('$message');</script>";
}
alert($message1);
alert($message2);
?>
This code will pop up a second alert message once you press the “ok” button on the first alert message.
Output:
Pop-Up Alert Message Using an Array or an Object
We can also use an array or an object instead of a variable to print in an alert box.
Example:
<?php
$alert = ["this", "is", "a", "demo", "warning"];
?>
<script>
var JavaScriptAlert = <?php echo json_encode($alert); ?>;
alert(JavaScriptAlert); // Your PHP alert!
</script>
json_encode()
is a built-in PHP function that converts the array or an object to a simple JSON
value.
Output:
The alert()
function is compatible with every major browser.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook