PHP 301 Redirect
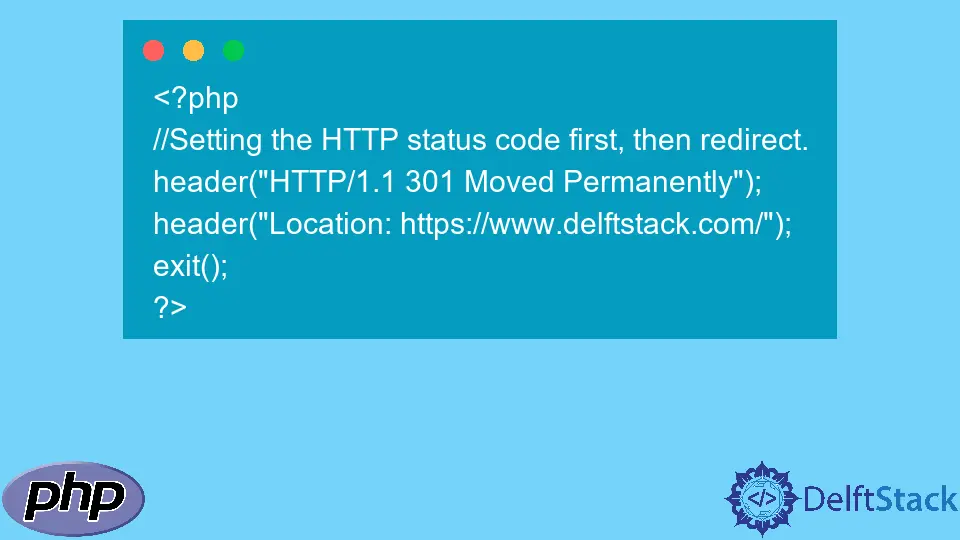
301 redirect is used to redirect a page permanently to a particular URL or page. Mostly used to improve SEO.
301 is the HTTP status code for a type of redirect.
In PHP, we can pass 301 as a parameter to PHP function header()
to redirect a page permanently.
This tutorial demonstrates how to redirect a page permanently using PHP and 301.
Use 301 and PHP header()
Function to Redirect to a Page or URL Permanently
We can use PHP header()
function and 301 in a few ways to permanently redirect to a given URL.
<?php
//Setting the HTTP status code first, then redirect.
header("HTTP/1.1 301 Moved Permanently");
header("Location: https://www.delftstack.com/");
exit();
?>
Here is a simple way:
<?php
//We can also pass the HTTP status code 301 as a parameter in PHP `header()`.
header("Location: https://www.delftstack.com/",TRUE,301);
?>
PHP also has a function to set HTTP status code http_response_code()
; we can use this function and then redirect, which will also be a 301 redirect. See example:
<?php
http_response_code(301);
header('Location: https://www.delftstack.com/');
exit;
?>
All three codes above have the same output, and it will permanently redirect the domain to our site https://www.delftstack.com/
.
First, we run index.php
with the above code, and it redirects to our site https://www.delftstack.com/
, then we comment the code in index.php
and run it again.
We will see the index.php
URL is permanently set to https://www.delftstack.com/
.
Output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook