How to Create a New Line in PHP
-
Use the
\n
Escape Sequence to Create a New Line in PHP -
Use the
\r\n
Escape Sequence to Create a New Line in PHP
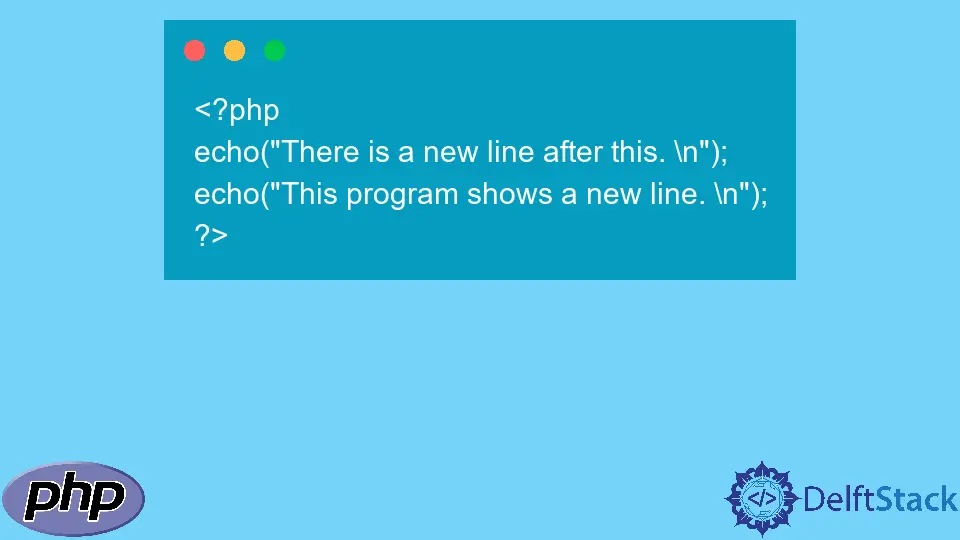
This article will introduce different methods to create a new line in PHP as you utilize escape sequences, such as \n
and \r\n
.
Use the \n
Escape Sequence to Create a New Line in PHP
In PHP, we have different methods to create a new line, and the simplest among those is by using the \n
escape sequence. Keep in mind that you need to use double quotes when including this escape sequence in your program. The correct syntax to execute the command is as follows:
echo("\n");
The program below shows how we can use the \n
escape sequence to create a new line in PHP.
<?php
echo("There is a new line after this. \n");
echo("This program shows a new line. \n");
?>
Output:
There is a new line after this.
This program shows a new line.
We can use the \n
function two times to create a line space between two text lines.
<?php
echo("There is a new line after this. \n\n");
echo("This program shows a new line. \n");
?>
Output:
There is a new line after this.
This program shows a new line.
Use the \r\n
Escape Sequence to Create a New Line in PHP
In PHP, we can also use the \r\n
escape sequence to create a new line; in addition, it also returns the carriage return, which resets the pointer and starts it from the left. The correct syntax to use it is as follows:
echo("\r\n");
Here’s the program that applies this method:
<?php
echo("There is a new line after this. \r\n");
echo("This program shows a new line. \n");
?>
Output:
There is a new line after this.
This program shows a new line.