How to Sort an Array of Associative Arrays by Value of a Given Key in PHP
-
Use
array_multisort()
Function to Sort an Array of Associative Arrays by the Value of a Given Key in PHP -
Use the
usort()
Function to Sort an Array of Associative Arrays by the Value of a Given Key in PHP
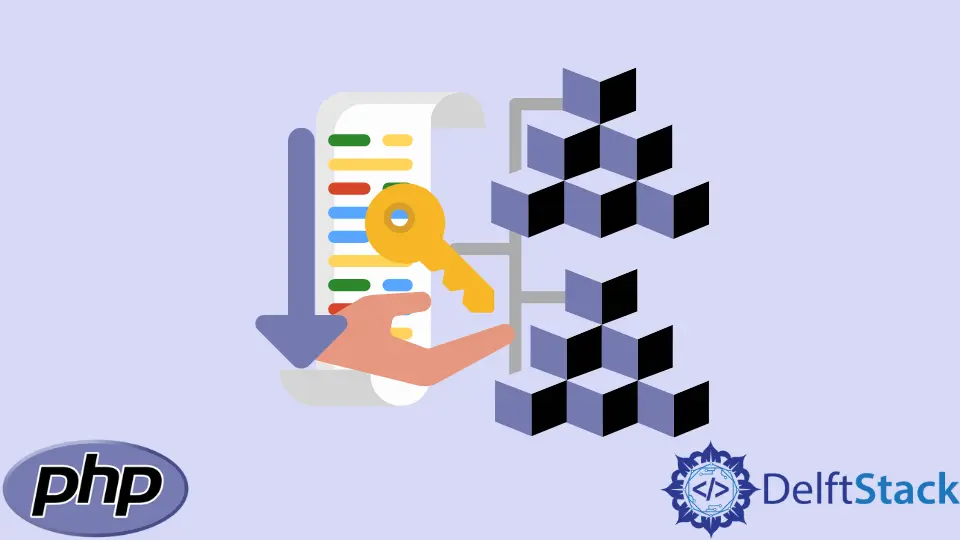
This article will introduce methods to sort an array of associative arrays by the value of a given key in PHP.
- Using
array_multisort()
function - Using
usort()
function
Use array_multisort()
Function to Sort an Array of Associative Arrays by the Value of a Given Key in PHP
We can use the built-in function array_multisort()
to sort an array of associative arrays by the value of a given key. It can sort many arrays at a time or a multidimensional array. The correct syntax to use this function is as follows
str_replace($arrayName, $sort_order, $sort_flags);
The built-in function array_multisort()
has three parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
$arrayName |
mandatory | It is the array that we want to sort. |
$sort_order |
optional | It specifies the order in which we will sort our array. It can be SORT_ASC for ascending order and SORT_DESC for descending order. Its default value is SORT_ASC . |
$sort_flags |
optional | It specifies the options for sorting. You can read it here. |
This function returns TRUE if it is successful. Otherwise, it returns FALSE.
The program below shows how we can use the array_multisort()
function to sort an array of associative arrays by the value of a given key.
<?php
$result = array(
array("firstname"=>"Olivia", "marks"=>85),
array("firstname"=>"Jacob", "marks"=>60),
array("firstname"=>"Henry", "marks"=>100),
array("firstname"=>"Lili", "marks"=>40),
array("firstname"=>"Stefan", "marks"=>5),
array("firstname"=>"Bonnie", "marks"=>97),
);
$marks = array();
foreach ($result as $key => $row)
{
$marks[$key] = $row['marks'];
}
array_multisort($marks, SORT_DESC, $result);
print_r($result);
?>
We have created an array $marks
containing the marks from the original array. After that, we have used the array_multisort()
function to sort $marks
array based on the $result
array. The output will be the $result
array sorted.
Output:
Array
(
[0] => Array
(
[firstname] => Henry
[marks] => 100
)
[1] => Array
(
[firstname] => Bonnie
[marks] => 97
)
[2] => Array
(
[firstname] => Olivia
[marks] => 85
)
[3] => Array
(
[firstname] => Jacob
[marks] => 60
)
[4] => Array
(
[firstname] => Lili
[marks] => 40
)
[5] => Array
(
[firstname] => Stefan
[marks] => 5
)
)
The array is sorted in descending order by the value of the marks key.
Use the usort()
Function to Sort an Array of Associative Arrays by the Value of a Given Key in PHP
In PHP, we can also use the usort()
function to sort an array of associative arrays by the value of a given key. This function sorts the array using a user-defined function. The correct syntax to use this function is as follows:
preg_replace($arrayName, $functionName);
The function the usort()
accepts two parameters. The detail of its parameters is as follows
Parameters | Description | |
---|---|---|
$arrayName |
mandatory | It is the array that we want to sort |
$functionName |
mandatory | It is the user-defined function that will sort the passed array. |
It returns TRUE if successful, FALSE otherwise. The program that sorts the array is as follows:
<?php
function DescSort($item1,$item2)
{
if ($item1['marks'] == $item2['marks']) return 0;
return ($item1['marks'] < $item2['marks']) ? 1 : -1;
}
$result = array(
array("firstname"=>"Olivia", "marks"=>85),
array("firstname"=>"Jacob", "marks"=>60),
array("firstname"=>"Henry", "marks"=>100),
array("firstname"=>"Lili", "marks"=>40),
array("firstname"=>"Stefan", "marks"=>5),
array("firstname"=>"Bonnie", "marks"=>97),
);
usort($result,'DescSort');
print_r($result);
?>
Output:
Array
(
[0] => Array
(
[firstname] => Henry
[marks] => 100
)
[1] => Array
(
[firstname] => Bonnie
[marks] => 97
)
[2] => Array
(
[firstname] => Olivia
[marks] => 85
)
[3] => Array
(
[firstname] => Jacob
[marks] => 60
)
[4] => Array
(
[firstname] => Lili
[marks] => 40
)
[5] => Array
(
[firstname] => Stefan
[marks] => 5
)
)
For ascending sort, the function will be:
<?php
function AscSort($item1,$item2)
{
if ($item1['marks'] == $item2['marks']) return 0;
return ($item1['marks'] > $item2['marks']) ? 1 : -1;
}
$result = array(
array("firstname"=>"Olivia", "marks"=>85),
array("firstname"=>"Jacob", "marks"=>60),
array("firstname"=>"Henry", "marks"=>100),
array("firstname"=>"Lili", "marks"=>40),
array("firstname"=>"Stefan", "marks"=>5),
array("firstname"=>"Bonnie", "marks"=>97),
);
usort($result,'AscSort');
print_r($result);
?>
Output:
Array
(
[0] => Array
(
[firstname] => Stefan
[marks] => 5
)
[1] => Array
(
[firstname] => Lili
[marks] => 40
)
[2] => Array
(
[firstname] => Jacob
[marks] => 60
)
[3] => Array
(
[firstname] => Olivia
[marks] => 85
)
[4] => Array
(
[firstname] => Bonnie
[marks] => 97
)
[5] => Array
(
[firstname] => Henry
[marks] => 100
)
)