How to Read a Large File Line by Line in PHP
-
Use
fgets()
Function to Read a Large File Line by Line in PHP -
Use
file()
Function to Read a Large File Line by Line in PHP -
Use
stream_get_line()
Function to Read a Large File Line by Line in PHP
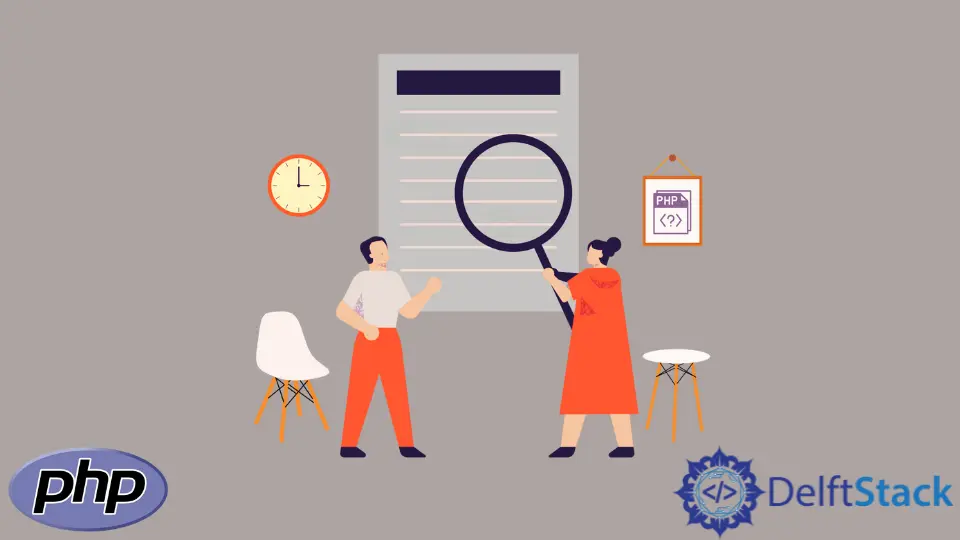
In this article, we will introduce methods to read a large file line by line in PHP.
- Using
fgets()
function - Using
file()
function - Using
stream_get_line()
function
Use fgets()
Function to Read a Large File Line by Line in PHP
The built-in function fgets()
reads a line from an open file. We can use this function to read a large file line by line. The correct syntax to use this function is as follows.
fgets($fileName, $lengthOfFile);
The function fgets()
has two parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
$fileName |
mandatory | It is the file that we want to read line by line. |
$lengthOfFile |
optional | It specifies the length of the file to be read. Its default value is 1024 bytes. |
This function returns a string
of lengthOfFile -1
from the file specified by the user if successful. It returns False
on failure.
The program below shows how we can use fgets()
function to read a large file line by line. We will use fopen()
and fclose()
functions to open and close a file respectively. We have a text file named flowers.txt
that contains the details of the flowers.
<?php
if ($file = fopen("flowers.txt", "r")) {
while(!feof($file)) {
$line = fgets($file);
echo ("$line");
}
fclose($file);
}
?>
We have used the feof()
function to read the file until the end. The output shows the content of the file read line by line.
Output:
Use file()
Function to Read a Large File Line by Line in PHP
In PHP, we can also use the file()
function to read a file line by line. The file()
function reads a file into an array line by line. The correct syntax to use this function is as follows:
file($fileName, $flags, $context)
The function file()
accepts three parameters. The detail of its parameters is as follows
Parameters | Description | |
---|---|---|
$fileName |
mandatory | It is the path where our file is stored. |
$flags |
optional | It specifies the constants that we can use as flags for this function. You can read it here. |
$context |
optional | It is the resource that you can create using stream_context_create() function. |
The program that reads the file using the file()
function is as follows:
<?php
foreach(file('flowers.txt') as $line) {
echo $line. "\n";
}
?>
Use stream_get_line()
Function to Read a Large File Line by Line in PHP
We can also use the stream_get_line()
function to read a file line by line. This function reads a file up to a delimiter. The correct syntax to use this function is as follows:
stream_get_line($handle, $length, $ending)
The function stream_get_line()
accepts three parameters. The detail of its parameters is as follows
Parameters | Description | |
---|---|---|
$handle |
mandatory | It is the path where our file is stored. |
$length |
mandatory | It specifies the file’s length to be read. |
$ending |
mandatory | It is a string that tells about the delimiter. |
The program that reads the file using stream_get_line()
function is as follows:
<?php
$file = fopen("flowers.txt", "r+");
while ($line = stream_get_line($file, 1024 * 1024, ".")) {
echo $line;
}
fclose($file);
?>
Output:
.
is removed from the output.