How to Parse a CSV File in PHP
Ralfh Bryan Perez
Feb 02, 2024
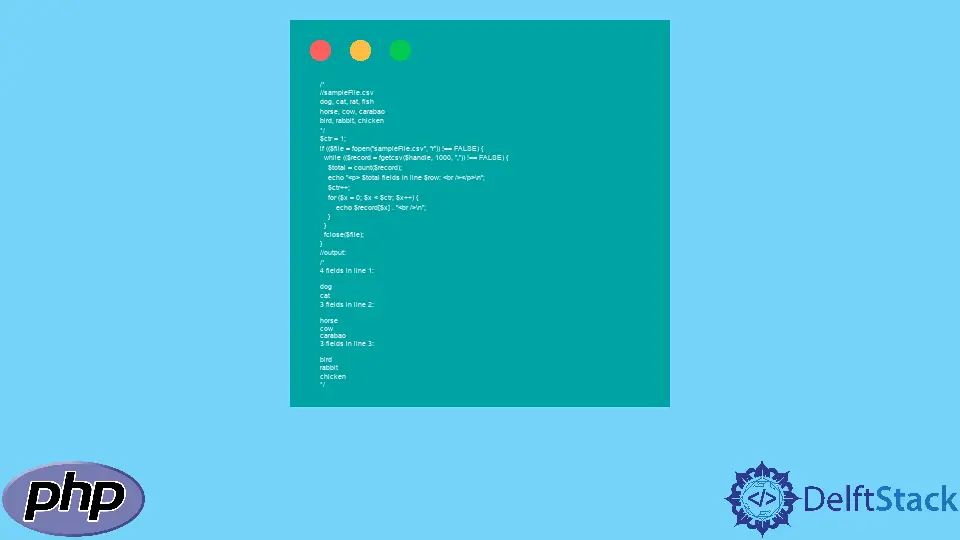
A CSV
is one type of file. It means Comma Separated Values
. It’s commonly used in different software such as Excel. This allows data to be saved in a tabular format and with a .csv
extension.
To parse a CSV
file in PHP, it can be done using the combination of fopen()
, fgetcsv()
and fclose()
functions that are built-in in PHP.
Example:
/*
//sampleFile.csv
dog, cat, rat, fish
horse, cow, carabao
bird, rabbit, chicken
*/
$ctr = 1;
if (($file = fopen("sampleFile.csv", "r")) !== FALSE) {
while (($record = fgetcsv($handle, 1000, ",")) !== FALSE) {
$total = count($record);
echo "<p> $total fields in line $row: <br /></p>\n";
$ctr++;
for ($x = 0; $x < $ctr; $x++) {
echo $record[$x] . "<br />\n";
}
}
fclose($file);
}
//output:
/*
4 fields in line 1:
dog
cat
3 fields in line 2:
horse
cow
carabao
3 fields in line 3:
bird
rabbit
chicken
*/
The example above parses the content of sampleFile.csv
using these functions:
fopen(Filename, Mode, Include_path, context)
This function accepts a URL or file, binds a named resource that is specified by filename to a stream.
Parameters:
Filename
(required) - This parameter specifies the file or URL to openMode
(required) - This represents the type of access you require to the file.Include_path
(optional) - Setting this parameter to1
will search for the file in theinclude_path
.context
(optional) - This specifies the content of the file handle. It’s a set of options that can be updated on the behavior of the stream.
fgetcsv(File, Length, Separator, Enclosure, Escape)
This function is responsible for parsing a line from an opened CSV file.
Parameters:
File
(required) - This parameter specifies the open file to return and parse a line from.Length
(optional on PHP 5 and below but required to PHP 5.1 and above) - This parameter is the maximum length of the line. This must be greater than the longest line in the file. If this parameter is not present, then the length is not limited, which makes it slower to parse.Separator
(optional) - This parameter is the field separator, and the default value is a comma -,
.Enclosure
(optional) - This parameter is the field enclosure, and the default value is"
.Escape
(optional) - This parameter is the escape character, and the default is\\
.
fclose()
This function closes an open file pointer.
Parameter:
File
(required) - This specifies what file to close.
Note
The file pointer must be valid, and must point to a file successfully opened by
fopen()
.