How to Get the Last Day of the Month With PHP Functions
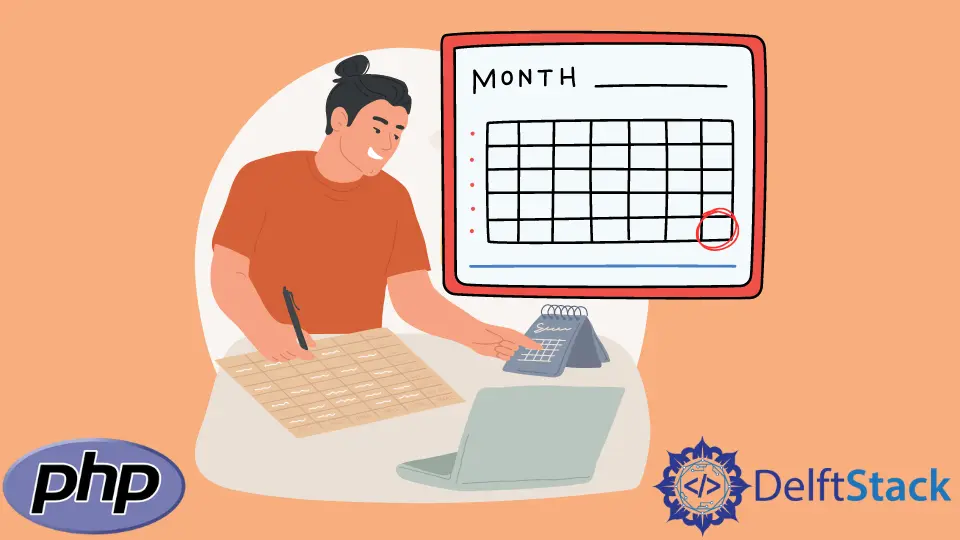
PHP has already built-in functions that will help in getting the last date of a given month. DateTime
and strtotime
functions are most useful in this scenario, though it’s well known and documented that strtotime
will not work accurately or give correct results after year 2038. However, strtotime
is awesome for converting dates.
Both functions use the format t
which according to the official manual returns the number of days in the month of a given date. Note the key to getting the last day of the month is the letter t
which returns the number of days in a given month.
PHP DateTime
to Get the Last Date of the Month
This is the best method to use to get the last date of a month both with future and past dates. The parameters passed in the format()
function is - Y
, m
and t
. These constants are defined in PHP.
A full numeric representation of a year, 4 digits is represented by letter Y
, for example, 2020
. Numeric representation of a month, with leading zeros, is by letter m
this is from 01
to 12
. And the number of days in the given month is represented by letter t
.
This is the best option if the PHP version used is after 5.3
$L = new DateTime( '2020-02-01' );
echo $L->format( 'Y-m-t' );
The code above supplies the DateTime
function with a date and using the format characters Y-m-t
to get the last date of the month on the date supplied.
PHP strtotime()
to Get Last Date of Month
strtotime()
is the best option for PHP version before 5.2.
$date = "2020-01-15";
echo date("Y-m-t", strtotime($date));