How to Get the First Element of an Array in PHP
- Use the Index of Elements to Get the First Element of an Array in PHP
-
Use the
reset()
Function to Get the First Element of an Array in PHP -
Use the
current()
Function to Get the First Element of an Array in PHP
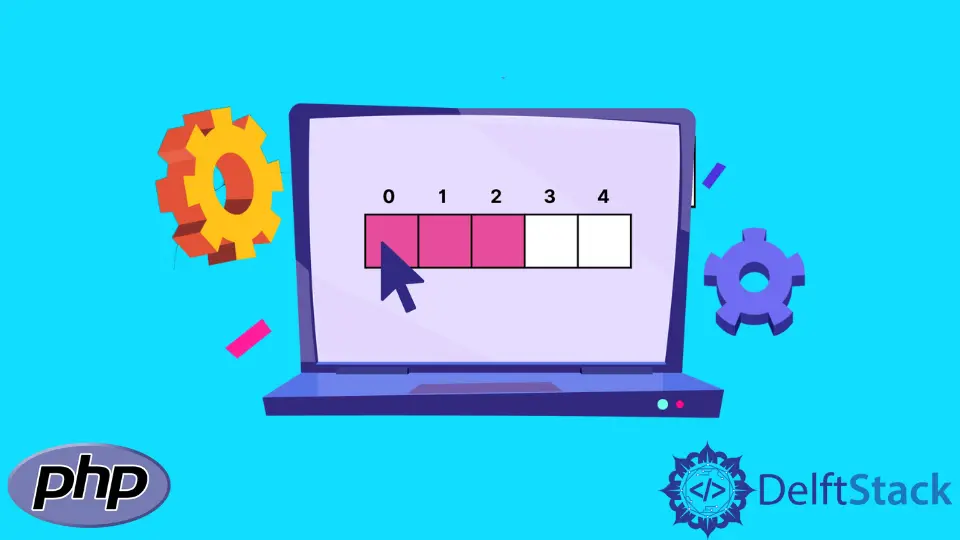
In this article, we will introduce methods to get the first element of an array in PHP.
- Using the index of the elements
- Using
reset()
function - Using
current()
function
Use the Index of Elements to Get the First Element of an Array in PHP
We know that the index of the first element in an array is 0, therefore, you can directly get the first element by accessing it through its index. The correct syntax to get the element via an index is as follows
$arrayName[0];
To get the first element, the index of the first element, 0
, is enclosed in square brackets along with the array name.
<?php
$flowers = array("Rose","Lili","Jasmine","Hibiscus","Tulip","Sun Flower","Daffodil","Daisy");
$firstElement = $flowers[0];
echo "The first element of the array is $firstElement."
?>
Output:
The first element of the array is Rose.
Use the reset()
Function to Get the First Element of an Array in PHP
The built-in function reset()
sets the pointer of an array to its start value i.e the first element of the array.
reset($arrayName);
It has only one parameter. It returns the value of the first element if the array is not empty. It returns false if the array is empty.
<?php
$flowers = array("Rose","Lili","Jasmine","Hibiscus","Tulip","Sun Flower","Daffodil","Daisy");
$firstElement = reset($flowers);
echo "The first element of the array is $firstElement."
?>
Output:
The first element of the array is Rose.
Use the current()
Function to Get the First Element of an Array in PHP
The current()
function is another built-in function used to get the value in the array that the pointer is currently pointing. It can be used to get the first element of the array if there is no internal pointer in the array. The pointer points to the first element by default. The correct syntax to use this function is as follows
current($arrayName);
It only accepts one parameter $arrayName
. The variable $arrayName
is an array of which we want to get the first element.
<?php
$flowers = array("Rose","Lili","Jasmine","Hibiscus","Tulip","Sun Flower","Daffodil","Daisy");
$firstElement = current($flowers);
echo "The first element of the array is $firstElement."
?>
Output:
The first element of the array is Rose.