How to Generate JSON File in PHP
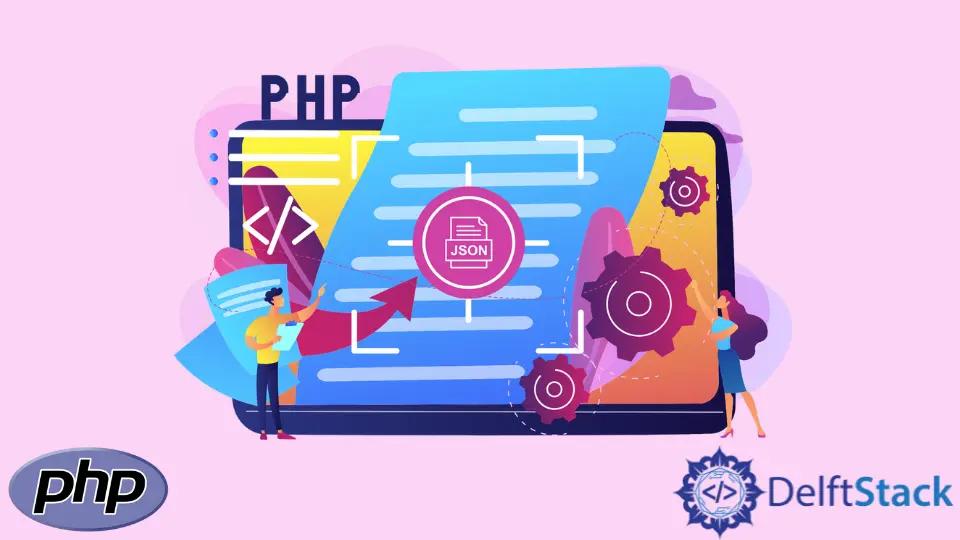
In this article, we will introduce the method to generate a .json
file in PHP.
- Using
file_put_contents()
function
Use file_put_contents()
Function to Generate a .Json File in PHP
The built-in function file_put_contents()
could write the content into a file in PHP. It searches for the file to write in, and if the desired file is not present, it creates a new file. We can use this function to create a .json
file. The correct syntax to use this function is as follows
file_get_contents($pathOfFile, $info, $customContext, $mode);
This function accepts four parameters. The detail of these parameters is as follows.
Parameter | Description | |
---|---|---|
$pathOfFile |
mandatory | It specifies the path of the file. |
$info |
mandatory | It specifies the information or data that you wish to write in a file. It can be a string. |
$customContext |
optional | It is used to specify a custom context. |
$mode |
optional | It specifies the mode in which the data will be written on the file. It can be FILE_USE_INCLUDE_PATH, FILE_APPEND, and, LOCK_EX. |
This function returns the number of bytes written on the file if successful and false otherwise.
The below program will create a new .json
file and store JSON
data into it
<?php
// data strored in array
$array = Array (
"0" => Array (
"id" => "01",
"name" => "Olivia Mason",
"designation" => "System Architect"
),
"1" => Array (
"id" => "02",
"name" => "Jennifer Laurence",
"designation" => "Senior Programmer"
),
"2" => Array (
"id" => "03",
"name" => "Medona Oliver",
"designation" => "Office Manager"
)
);
// encode array to json
$json = json_encode($array);
$bytes = file_put_contents("myfile.json", $json);
echo "The number of bytes written are $bytes.";
?>
We use json_encode()
function to convert the data stored in the array to a JSON
string. Once the data is converted to a JSON
string, file_put_contents()
function creates a .json
file and writes data into it. The output shows the number of bytes, which means the data is written successfully.
Output:
The number of bytes written is 207.