How to Convert an Integer Into a String in PHP
- Use Inline Variable Parsing to Convert an Integer to a String in PHP
-
Use
strval()
Function to Convert an Integer to a String in PHP - Use Explicit Casting to Convert an Integer to a String in PHP
- Use String Concatenation to Implicitly Convert an Integer to a String in PHP
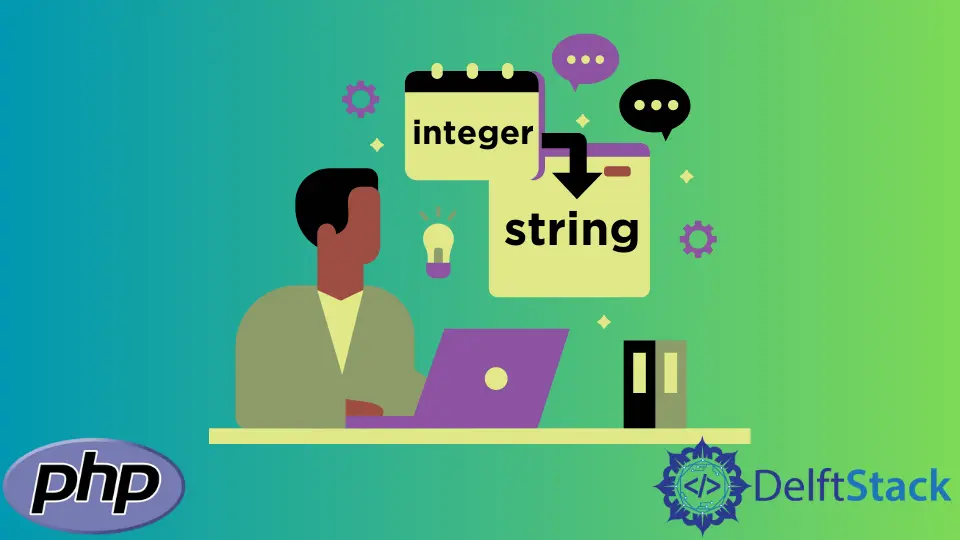
In this article, we will introduce methods to convert an integer to a string.
- Using inline variable parsing
- Using
strval()
function - By explicit type casting
- Using PHP string concatenation
Use Inline Variable Parsing to Convert an Integer to a String in PHP
When an integer
is used inside a string
to display the string
, it is already converted to a string
before display.
$integer = 5;
echo "The string is $integer";
The variable $integer
is used in a string to display its value. It is converted to the string first.
<?php
$variable = 10;
echo "The variable is converted to a string and its value is $variable.";
?>
The output is a string
that shows the integer
value converted to the string
.
Output:
The variable is converted to a string and its value is 10.
Use strval()
Function to Convert an Integer to a String in PHP
The function strval()
is a built-in function in PHP to convert any type of variable to a string
. The variable is passed as a parameter.
strval($variableName);
The $variableName
variable shows the value that we want to convert to a string. After executing it, it returns the variable as a string
.
<?php
$variable = 10;
$string1 = strval($variable);
echo "The variable is converted to a string and its value is $string1.";
?>
strval()
cannot be used for an array or an object. If so, the function returns the type name of the parameter passed.Output:
The variable is converted to a string and its value is 10.
Use Explicit Casting to Convert an Integer to a String in PHP
In explicit casting, we convert a variable on a particular data type to another data type manually. We will use explicit casting to convert an integer
to a string
.
$string = (string)$intVariable
The variable string will contain the casted value of $intVariable
.
<?php
$variable = 10;
$string1 = (string)$variable;
echo "The variable is converted to a string and its value is $string1.";
?>
This is a useful way of converting an integer
to a string
in PHP. In the first method, implicit casting has been implemented.
Output:
The variable is converted to a string and its value is 10.
Use String Concatenation to Implicitly Convert an Integer to a String in PHP
To join two strings together, we used string concatenation. We can also use string concatenation to convert an integer
to a string
.
"First string".$variablename."Second string"
The string concatenation will result in a string
that contains the integer
being converted to the string
.
<?php
$variable = 10;
$string1 = "The variable is converted to a string and its value is ".$variable.".";
echo "$string1";
?>
We have stored the result of string concatenation in a new string
variable and then displayed it.
Output:
The variable is converted to a string and its value is 10.
If you only need to convert the integer
to string
, then you simply put empty string ""
before or after the integer
.
<?php
$variable = 10;
$string1 = "".$variable;
echo "$string1";
$string1 = $variable."";
echo "$string1";
?>