How to Convert a Timestamp to a Readable Date or Time in PHP
-
Use
date()
Function to Convert a Timestamp to a Date/Time in PHP -
Use
setTimestamp()
Function to Convert a Timestamp to a Date in PHP -
Use
createFromFormat()
Function to Convert a Timestamp to a Date in PHP
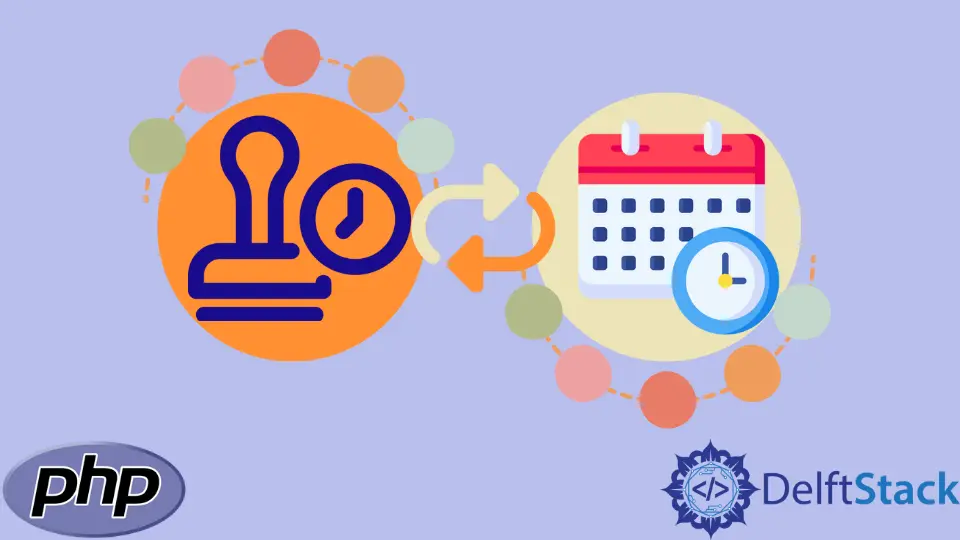
In this article, we will introduce methods to convert a timestamp
to date
in PHP.
- Using
date()
function - Using
setTimestamp()
function - Using
createFromFormat()
function
Use date()
Function to Convert a Timestamp to a Date/Time in PHP
The date()
function converts a timestamp
to a human readable date
or time
. The correct syntax to use this function is as follows
date($format, $timestamp);
It has two parameters. The parameter $format
is the date-time format that the timestamp is converted to. The other parameter $timestamp
is an optional parameter. It gives the date according to the timestamp
passed. If it is omitted, it uses the current date by default.
<?php
$date = date('d-m-Y H:i:s', 1565600000);
echo "The date is $date.";
?>
The date format here is day-month-year
, and the time format is hour:minute:second
.
Output:
The date and time are 12-08-2019 08:53:20.
Use setTimestamp()
Function to Convert a Timestamp to a Date in PHP
The built-in setTimestamp()
converts the given timestamp
to date
or time
. To set the format of the date we will use format()
function.
$datetimeObject->setTimestamp($timestamp);
Example Codes:
<?php
$date = new DateTime();
$date->setTimestamp(1565600000);
$variable = $date->format('U = d-m-Y H:i:s');
echo "The date and time is $variable.";
?>
Output:
The date and time are 1565600000 = 12-08-2019 08:53:20.
Use createFromFormat()
Function to Convert a Timestamp to a Date in PHP
The built-in function createFromFormat()
gets the date
by passing the timestamp
as a parameter to this function.
DateTime::createFromFormat($format, $time, $timezone);
The variable $format
is the format of the date, $time
is the time given in string and $timezone
tells about the time zone. The first two parameters are the mandatory parameters.
<?php
// Calling the createFromFormat() function
$datetime = DateTime::createFromFormat('U', '1565600000');
// Getting the new formatted datetime
$date= $datetime->format('d-m-Y H:i:s');
echo "The date and time is $date.";
?>
The format "d-m-Y H:i:s"
displays both date
and time
.
Output:
The date and time are 12-08-2019 08:53:20.