How to Add Days to Date in PHP
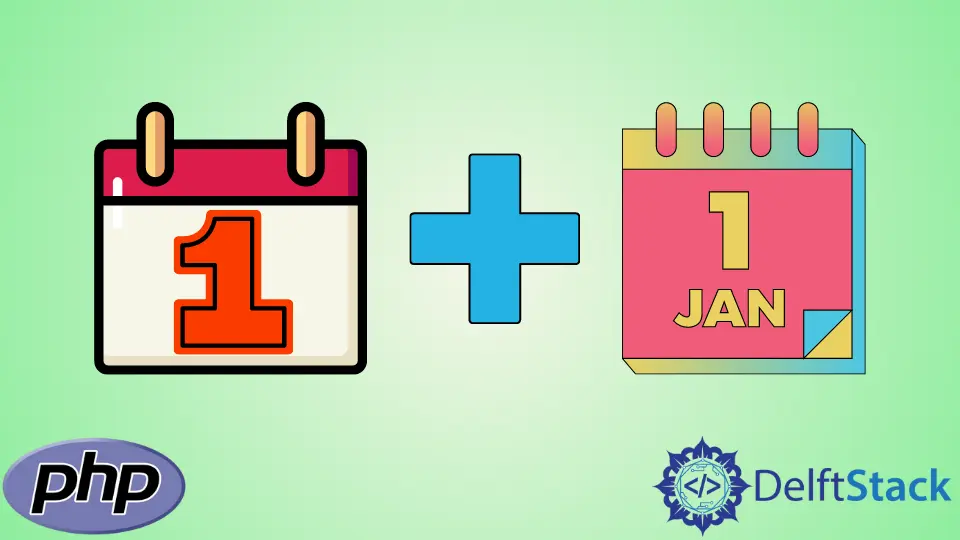
Manipulating date
string in PHP can be done in many ways, can add or deduct hours, months, years, etc. PHP provides different functions such as DateTime
, date_add
and combination of strtotime()
and date()
.
add
Method in DateTime()
to Add Days in PHP
Using PHP version 5.3 and above, DateTime
object and it’s add
method can also be a solution. DateTime
supports more date formats than strtotime
and date
. Using an object also easier than arbitrary functions. For example, when comparing two dates, it’s direct in DateTime
, but in strtotime
, converting the date first to timestamp is necessary.
Example:
<?php
$oldDate = "2020-02-27";
$newDate = new DateTime($oldDate);
$newDate->add(new DateInterval('P1D')); // P1D means a period of 1 day
$fomattedDate = $date->format('Y-m-d');
?>
The following code will print output:
echo $fomattedDate; //output: 2020-02-28
The hard part of using DateTime()
is the DateInterval
object. This accepts a valid interval specification. The proper format starts with the letter P
which means period
, followed by an integer value, then D
for the day. If the duration is time, the last part needs to be T
.
Combination of strtotime()
and date()
to Add Days to Date in PHP
The strtotime()
is a PHP function which is used to convert an English textual date description to a UNIX timestamp. The strtotime
function will accept a string value that represents date-time.
Example:
<?php
$oldDate = "2020-02-27";
$date1 = date("Y-m-d", strtotime($oldDate.'+ 1 days'));
$date2 = date("Y-m-d", strtotime($oldDate.'+ 2 days'));
?>
The following code will output:
echo $date1; //output: 2020-02-28
echo $date2; //output: 2020-02-29
The above example will accept a date string with proper format and return the new date using strtotime
and +1 days
. date()
function gave the proper format.
Note: To avoid obscurity, it’s recommended to use ISO 8601 (YYYY-MM-DD)
format.
date_add()
to Add Days to Date in PHP
This approach is the easiest among the others to add days, months, years, hours, minutes and seconds.
Example:
$oldDate = date_create("2020-02-27");
date_add($oldDate, date_interval_create_from_date_string("1 day"));
In application:
echo date_format($date,"Y-m-d"); //output: 2020-02-28
The above example also add dates to a date string, date_create()
creates a DateTime object. date_interval_create_from_date_string()
sets up a DateInterval
from the parts of the string. Then finally, date_add()
incrementes the date value.