How to Hash and Verify Password With Php_hash Method
-
Hash Passwords Using the Default Algorithm With
PASSWORD_DEFAULT
Constant in PHP -
Hash Passwords Using an Explicit Algorithm Using
PASSWORD_BCRYPT
Constant in PHP -
Hash Passwords Using the Explicit Algorithm With
PASSWORD_BCRYPT
Constant and Option Parameter in PHP
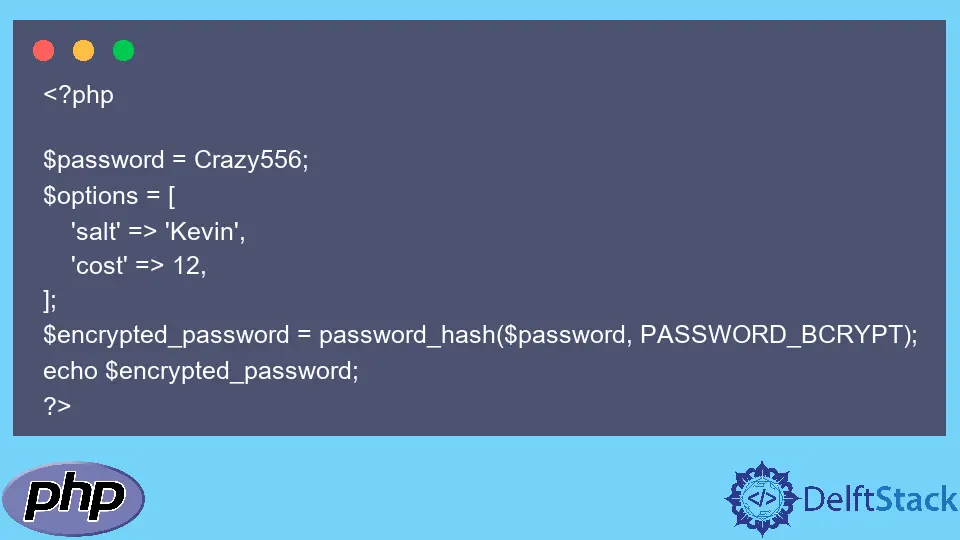
We will look at how to hash a password in PHP using the default PASSWORD_DEFAULT
Constant that uses the default algorithm.
We will also look at how to hash a password in PHP using the PASSWORD_BCRYPT
Constant that specifies the explicit algorithm as bcrypt
and using PASSWORD_BCRYPT
Constant and the option
parameter that allows one to specify the algorithm count and salt.
Hash Passwords Using the Default Algorithm With PASSWORD_DEFAULT
Constant in PHP
We will create a hashed password using the default algorithm by specifying PASSWORD_DEFAULT
in the password_hash
method.
<?php
$password = Crazy556;
$encrypted_password = password_hash($password, PASSWORD_DEFAULT);
echo $encrypted_password;
?>
Output:
$2y$10$bPtyWBeqYSa3HilGaTLB1uOV6jPt0fbZwxmzexXWQ3RKb8BeL3VOW
Hash Passwords Using an Explicit Algorithm Using PASSWORD_BCRYPT
Constant in PHP
We will create a hashed password using the bcrypt
algorithm by specifying PASSWORD_BCRYPT
in the password_hash
method.
<?php
$password = Crazy556;
$encrypted_password = password_hash($password, PASSWORD_BCRYPT);
echo $encrypted_password;
?>
Output:
$2y$10$bPtyWBeqYSa3HilGaTLB1uOV6jPt0fbZwxmzexXWQ3RKb8BeL3VOW
Hash Passwords Using the Explicit Algorithm With PASSWORD_BCRYPT
Constant and Option Parameter in PHP
We will create a hashed password using the bcrypt
algorithm by specifying the PASSWORD_BCRYPT
constant in the password_hash
method and specifying the salt
and cost
strings in the options
parameter.
<?php
$password = Crazy556;
$options = [
'salt' => 'Kevin',
'cost' => 12,
];
$encrypted_password = password_hash($password, PASSWORD_BCRYPT);
echo $encrypted_password;
?>
Output:
$2y$10$TTiV87qqyNNIcQeihPl85ei42pyBv.MhZoJ4sNFva.yK4U09iUB5G