How to Get Time Zone in PHP
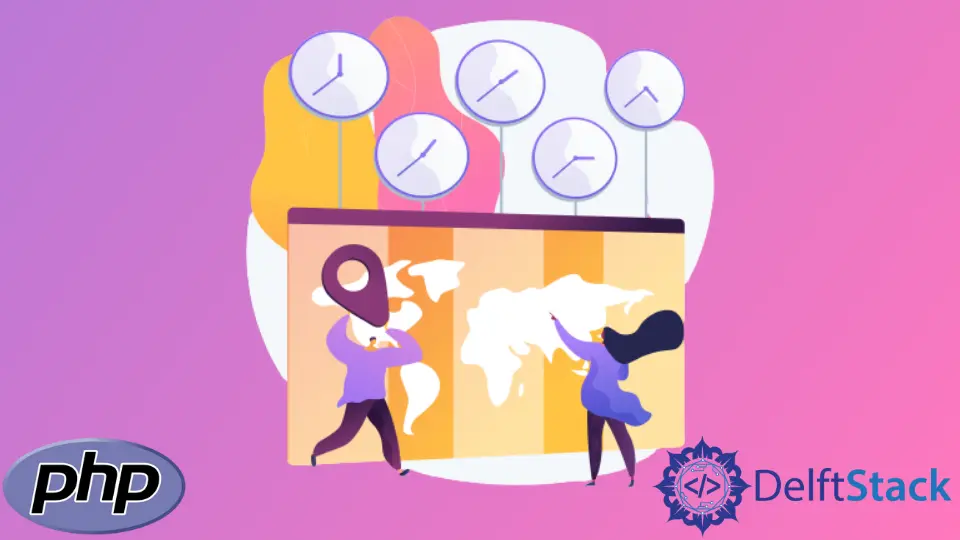
This article will introduce the method to get the time zone in PHP.
Get Time Zone in PHP
When working on a large-scale PHP application in which users from all over the world are accessing and entering data, we need to create a time zone and set a standard date and time for all users. This is to easily convert the date and time according to users’ time zone.
There are a few built-in functions of PHP that we can use to get and set time zone. We can call this built-in PHP function to get the default time zone.
# php
echo date_default_timezone_get();
It will get the name of the default time zone, as shown below.
Output:
The default time zone is UTC from the above results, but we can easily change it using another PHP built-in function. So, let’s change our default time zone as shown below.
# php
date_default_timezone_set("Europe/Amsterdam");
echo date_default_timezone_get();
Output:
Our default time zone was set to UTC from the above example, but we changed it to Europe/Amsterdam using PHP’s built-in function.
Another built-in function in PHP is used to get the time zone of the given DateTime
object. If we have a DateTime
object for which we want to find the time zone, we can quickly get it using the following function.
# php
$date=date_create(null,timezone_open("Europe/Amsterdam"));
$timezone=date_timezone_get($date);
echo timezone_name_get($timezone);
Output:
As you can see, we created a new date with the time zone Europe/Amsterdam. With the help of the built-in function of PHP, we were able to extract the time zone from that DateTime
object.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn