How to Get the Current Month of a Date in PHP
-
Using the
date()
Function to Get the Current Month of a Date in PHP -
Using
strtotime()
anddate()
Functions to Get the Current Month of a Date in PHP -
Get the Current Month by Using the
DateTime
Class in PHP
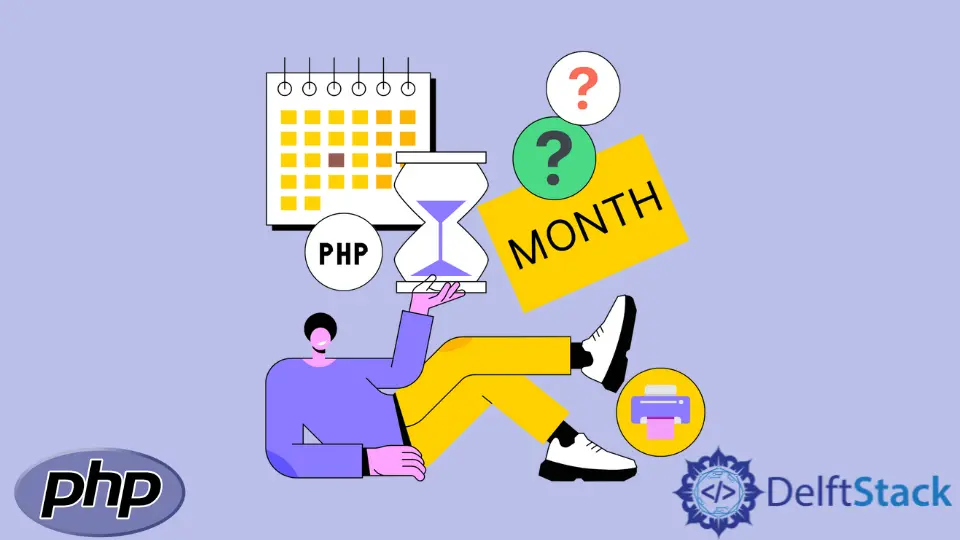
The date()
function is a built-in PHP function that formats the timestamp. In UNIX Timestamp, the computer saves the date and time. Since January 1, 1970, this time has been measured in seconds. Because this is difficult for humans to comprehend, PHP changes the timestamp to a more legible and intelligible format.
There are several methods to get the month portion of a date in PHP. In the following sections, you’ll learn how to get the month of date from the current date or any date.
Using the date()
Function to Get the Current Month of a Date in PHP
PHP’s date()
function can provide you with the date and time-related information based on the formatting characters in its first parameter. A maximum of two arguments can be sent to the function. It will return information about the current time if you just use one argument.
To generate three distinct forms of a month, use three different formatting characters in the first parameter of the date()
function. These are the formatting characters:
The date()
function has the following formatting options: The date()
function’s format parameter is a string that can include several characters, allowing you to produce dates in a variety of forms, as seen below:
<?php
echo "Current month representation, having leading zero in 2 digit is: " . date("m");
echo "\n";
echo "The Syntex representation of current month with leading zero is: " . date("M");
echo "\n";
echo "Current month representation,not having leading zero in 2 digit is: " . date("n");
?>
Output:
Current month representation, having leading zero in 2 digits is: 12
The Syntex representation of the current month with leading zero is: Dec
Current month representation, not having leading zero in 2 digits is: 12
Here,
d
– Represents the month’s day. Two numerals with leading zeros are used (01 or 31).
D
- In the text, represents the day of the week (Mon
to Sun
).
m
- The month is represented by the letter m in numerals with leading zeros (01 or 12).
M
- In the text, M
stands for month and is shortened (Jan
to Dec
).
y
– Denotes a two-digit year (07 or 21).
Y
- Year in four numbers is represented by the letter Y.
Using strtotime()
and date()
Functions to Get the Current Month of a Date in PHP
We’ll go through two steps to get the month from any date using the strtotime()
method.
To begin, transform a date to its timestamp equal. Use the date()
function with the formatting character to get the month from that timestamp.
<?php
$timestamp = strtotime("5th September 2003");
echo "Current month representation, having leading zero in 2 digits is: " . date("m", $timestamp);
echo "\n";
echo "The Syntex representation of current month with leading zero is: " . date("M", $timestamp);
echo "\n";
echo "Current month representation,not having leading zero in 2 digits is: " . date("n", $timestamp);
?>
Output:
Current month representation, having leading zero in 2 digits is: 09
The Syntex representation of the current month with leading zero is: Sep
Current month representation,not having leading zero in 2 digits is: 9
Get the Current Month by Using the DateTime
Class in PHP
PHP 5.2 introduces certain pre-built classes to assist developers in solving common problems. DateTime is one of the classes, and it deals with date and time difficulties. Follow these two steps to retrieve the current month using the DateTime class:
First, create a DateTime()
class object. The current time is represented when the DateTime()
class is used without any parameters.
Then, use the DateTime()
class’s format()
function to get the year from the newly formed object.
<?php
$now = new DateTime();
echo "Current month representation, having leading zero in 2 digit is: " . $now->format('m');
echo "\n";
echo "The Syntex representation of current month with leading zero is: " . $now->format('M');
echo "\n";
echo "Current month representation,not having leading zero in 2 digit is: " . $now->format('n');
?>
Output:
The 2 digit representation of the current month with leading zero is: 12
The textual representation of the current month with leading zero is: Dec
The 2 digit representation of the current month without leading zero is: 12