How to Get Key From a PHP Array
-
Get Keys From a PHP Array Using the
array_key
Method -
Get Keys From a PHP Array Using the
array_search
Method -
Get Keys From a PHP Array Using the
foreach
Loop -
Get Keys From a PHP Array Using the
while
Loop and thekey
Method -
Get Keys From a PHP Array Using Combination of the
foreach
Loop andarray_key
Method
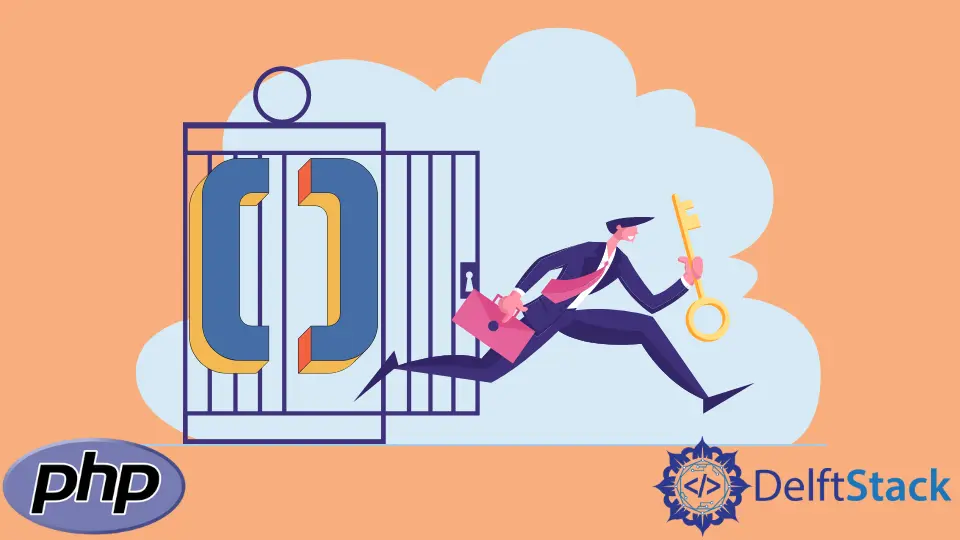
We will look at how to get keys from a PHP array using the array_key
and array_search
methods.
We will also look at how to get keys from a PHP array using the foreach
loop, using the while
loop and the key
method, and using a combination of the foreach
loop and the array_key
method.
Get Keys From a PHP Array Using the array_key
Method
We will create an array with the corresponding key-value pairs and fetch the keys with the array_keys
method.
<?php
$profile=array("Name"=>"Kevin","Occupation"=>"Programmer","Hobby"=>"Reading");
print_r(array_keys($profile));
?>
Output:
Array ( [0] => Name [1] => Occupation [2] => Hobby )
Get Keys From a PHP Array Using the array_search
Method
We will create an array with the corresponding key-value pairs and fetch the keys with the array_search
method.
<?php
$profile=array("Name"=>"Kevin","Occupation"=>"Programmer","Hobby"=>"Reading");
$key1 = array_search('Kevin', $profile);
$key2 = array_search('Programmer', $profile);
print_r([$key1,$key2])
?>
Output:
Array ( [0] => Name [1] => Occupation )
Get Keys From a PHP Array Using the foreach
Loop
We will create an array with the corresponding key-value pairs and fetch the keys with the foreach
loop.
<?php
$profile=array("Name"=>"Kevin","Occupation"=>"Programmer","Hobby"=>"Reading");
foreach($profile as $key => $value) {
echo $key."\n";
}
?>
Output:
Name
Occupation
Hobby
Get Keys From a PHP Array Using the while
Loop and the key
Method
We will create an array with the corresponding key-value pairs and fetch the keys with the while
loop and the key
method.
<?php
$profile=array("Name"=>"Kevin","Occupation"=>"Programmer","Hobby"=>"Reading");
while($element = current($profile)) {
echo key($profile)."\n";
next($profile);
}
?>
Output:
Name
Occupation
Hobby
Get Keys From a PHP Array Using Combination of the foreach
Loop and array_key
Method
We will create an array with the corresponding key-value pairs and fetch the keys using a combination of the foreach
loop and array_key
method.
<?php
$profile=array("Name"=>"Kevin","Occupation"=>"Programmer","Hobby"=>"Reading");
foreach(array_keys($profile) as $key)
{
echo $key."\n";
}
?>
Output:
Name
Occupation
Hobby