die() and exit() Functions in PHP
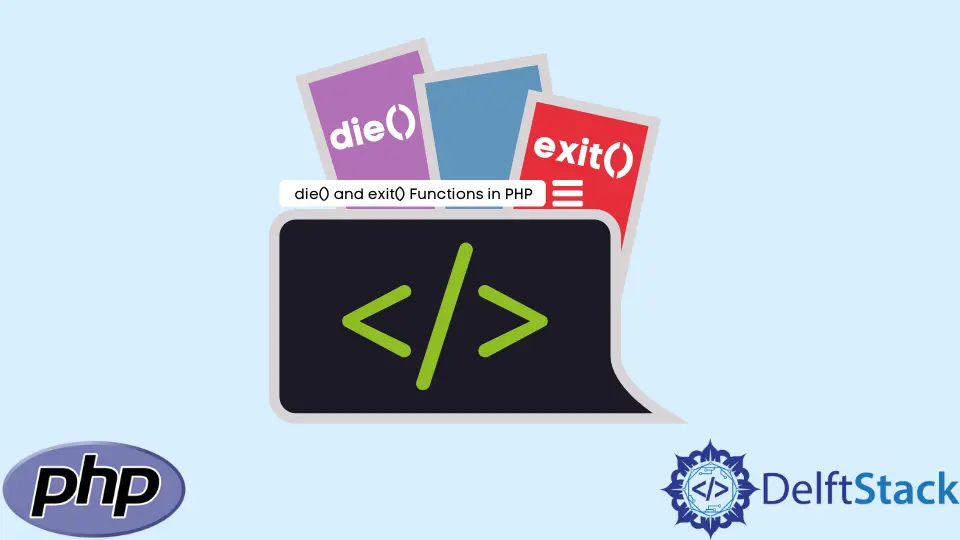
The die()
and exit()
functions in PHP have the same purpose. The language constructs exit()
and die()
both output a message and end the current PHP script.
This tutorial will look at the differences between PHP’s die()
and exit()
functions.
Using the exit()
Function in PHP
The exit()
is a built-in function that prints a message and exits a PHP script. It’s ideal for terminating an execution due to an error.
Syntax:
exit("Type a Message Here");
or
exit();
The example code below illustrates how you can end an execution using the exit()
function.
Code Snippet:
<?php
$s = 300;
$v = 300.1;
if($s===$v){
exit('The two are equal');
}else{
exit ('The two are not equal');
}
?>
Output:
The two are not equal
The exit()
function exits the script and prints the message The two are not equal
.
Using the die()
Function in PHP
The die()
function works like the exit()
function. We can use the die()
function to check for errors and stop execution.
The example below illustrates how to utilize the die()
function to end the execution of a database connection if an error occurs.
Code Snippet:
<?php
$user = 'root';
$pass = '';
$db = 'sample tutorial';
$con = mysqli_connect("localhost", $user, $pass, $db);
if ($con->connect_error) {
die("Connection failed: " . $con->connect_error);
}
?>
The code above does not have an output. If the database connection is unsuccessful, then the die()
function will end the process.
If the connection is successful, the die()
function can throw in an exception, and the function will not end the process.
exit() |
die() |
---|---|
Exits the process without exceptions | Can throw in an exception |
Exit script and prints message | Prints a message and end a process |
Originates in C | Originates from Perl |
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn