How to Determine Referer in PHP
- Understanding the HTTP Referer
- Storing User Sessions
- Displaying the Last Visited Server Location
- Security Considerations
- Conclusion
- FAQ
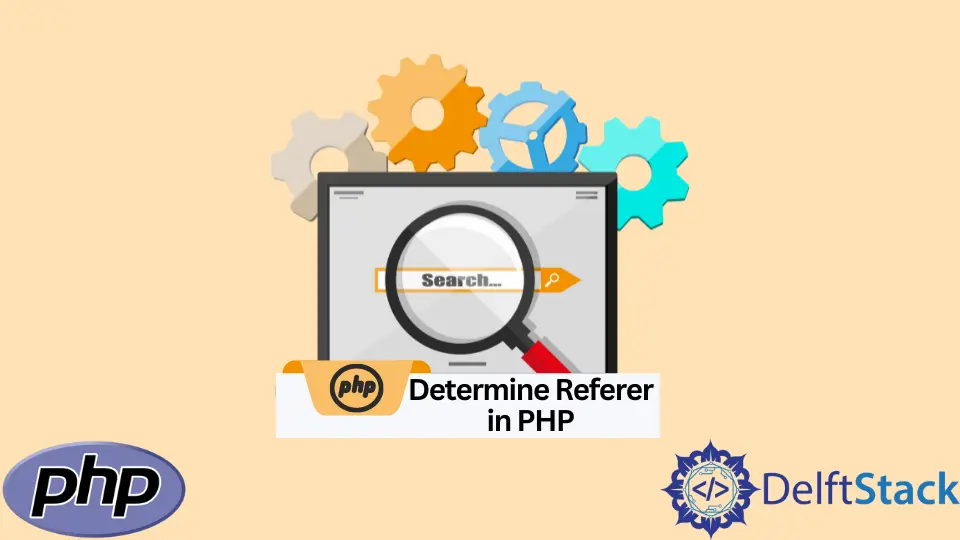
Determining the referer in PHP can be crucial for understanding user navigation and enhancing your web application’s functionality.
In this tutorial, we will explore a straightforward yet secure way to determine the referer using PHP. By storing user sessions, we can track the first and subsequent pages visited. Additionally, we will utilize the HTTP referer header to show the last visited server location. Whether you’re building a web application or simply curious about user behavior, this guide will provide you with the insights you need to effectively manage referer information in your PHP projects.
Understanding the HTTP Referer
The HTTP referer is an HTTP header field that identifies the address of the webpage that linked to the resource being requested. In simpler terms, it tells you where the user came from before arriving at your page. This information can be incredibly useful for analytics, security checks, and user experience improvements.
In PHP, you can access the referer using the $_SERVER
superglobal array. The $_SERVER['HTTP_REFERER']
variable contains the URL of the referring page. However, it’s important to note that this value can be manipulated, so it should not be solely relied upon for security purposes.
Storing User Sessions
To effectively determine the referer, you can leverage PHP sessions. Sessions allow you to store user-specific information across multiple pages. Here’s how you can set up user sessions to track the referer.
First, ensure that sessions are started at the beginning of your script. Then, you can check if a session variable for the referer is already set. If it is not, you can assign the current referer to it.
Here’s a simple implementation:
session_start();
if (!isset($_SESSION['referer'])) {
$_SESSION['referer'] = isset($_SERVER['HTTP_REFERER']) ? $_SERVER['HTTP_REFERER'] : 'Direct Visit';
}
Output:
Referer stored in session
This code snippet starts a session and checks if the ‘referer’ session variable is set. If it isn’t, it assigns the value of $_SERVER['HTTP_REFERER']
to it. If the referer is not available (like in a direct visit), it defaults to ‘Direct Visit’. This way, you can keep track of the user’s journey through your website.
Displaying the Last Visited Server Location
Once you have stored the referer in the session, you can easily display it on your webpage. This can help you understand where your traffic is coming from and improve your marketing strategies.
To achieve this, you can simply echo the session variable on your page. Here’s how:
session_start();
if (isset($_SESSION['referer'])) {
echo "Last visited server location: " . htmlspecialchars($_SESSION['referer']);
} else {
echo "No referer information available.";
}
Output:
Last visited server location: [Referer URL]
In this code, we start the session again and check if the ‘referer’ variable exists. If it does, we display it using htmlspecialchars()
to prevent XSS attacks. If the variable is not set, we inform the user that no referer information is available. This simple implementation enhances user experience by providing relevant information.
Security Considerations
While tracking referers can provide valuable insights, it’s essential to approach this with caution. The HTTP referer can be easily spoofed, meaning it cannot be trusted entirely for security-sensitive operations. Always validate and sanitize the input before using it in your application.
Additionally, consider implementing measures to protect against CSRF attacks, especially if you are using the referer to determine access to certain resources. Using tokens and validating user actions can help mitigate these risks.
Conclusion
In this tutorial, we explored how to determine the referer in PHP by utilizing sessions and the HTTP referer header. By storing user sessions, we can track where users are coming from and enhance our web applications accordingly. Remember to always consider security implications when handling referer data. By following the methods outlined above, you can effectively manage referer information and improve your website’s user experience.
FAQ
-
What is the HTTP referer?
The HTTP referer is an HTTP header that indicates the URL of the webpage that linked to the resource being requested. -
How do I access the referer in PHP?
You can access the referer in PHP using the$_SERVER['HTTP_REFERER']
variable. -
Can the HTTP referer be trusted for security?
No, the HTTP referer can be easily spoofed, so it should not be solely relied upon for security purposes. -
How can I store referer information in PHP?
You can store referer information in PHP using sessions by starting a session and assigning the referer value to a session variable. -
What should I do if the referer is not available?
If the referer is not available, you can default to a message like ‘Direct Visit’ to indicate that the user arrived directly without a prior page.
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn