How to Convert PHP Array to Javascript JSON
Kevin Amayi
Feb 02, 2024
-
Convert PHP to Javascript
JSON
Usingjson_encode
Method -
Convert PHP to Javascript Array Using the PHP
implode
Method -
Convert PHP Into Javascript Array Using the PHP
implode
Method Inside Javascript Array - Use PHP Directly in Javascript
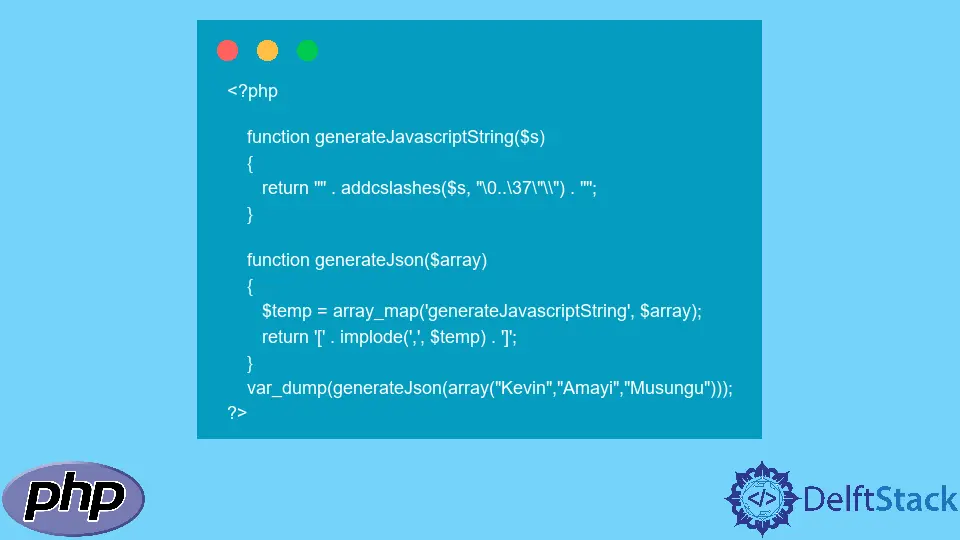
We will introduce how to convert PHP into Javascript JSON
using the json_encode
Method.
Save the below code in the file filename.php
. You will need to run this code from a server like Apache
with PHP installed.
Convert PHP to Javascript JSON
Using json_encode
Method
<?php
$ages = array("Kevin"=>24, "Donald"=>23, "Alvin"=>27);
echo json_encode($ages);
?>
Output:
{"Kevin":24,"Donald":23,"Alvin":27}
Convert PHP to Javascript Array Using the PHP implode
Method
<?php
function generateJavascriptString($s)
{
return '"' . addcslashes($s, "\0..\37\"\\") . '"';
}
function generateJson($array)
{
$temp = array_map('generateJavascriptString', $array);
return '[' . implode(',', $temp) . ']';
}
var_dump(generateJson(array("Kevin","Amayi","Musungu")));
?>
Output:
"["Kevin","Amayi","Musungu"]"
Convert PHP Into Javascript Array Using the PHP implode
Method Inside Javascript Array
<script>
var js_array = [<?php echo '"'.implode('","',['Programming', 'Coding']).'"' ?>];
console.log(js_array);
</script>
Output:
Array["Programmig","Coding"]
Use PHP Directly in Javascript
<!DOCTYPE html>
<html>
<body>
<?php
$sevenMultiples =array(7,14,28,35,42,49);
?>
<script type="text/javascript">
var jsArray = new Array();
<?php
$seveMultipleArray=count($sevenMultiples);
for($i=0;$i<$seveMultipleArray;$i++)
{
echo "jsArray[$i] = ". $sevenMultiples[$i] . ";\n";
}
?>
console.log(jsArray);
</script>
</body>
</html>
Output:
Array(6) [ 7, 14, 28, 35, 42, 49 ]