How to Convert Object to String in PHP
-
Use the
__toString()
Magic Method to Convert an Object to a String in PHP -
Use the
serialize()
Function to Convert an Object to a String in PHP -
Use the
print_r()
Function to Convert an Object to a Strig in PHP
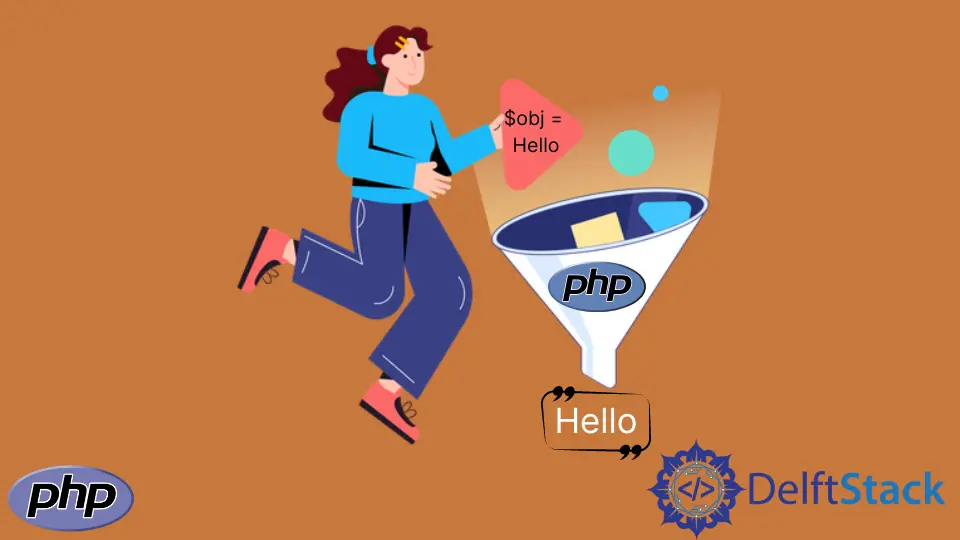
This tutorial introduces different methods to convert an object to a string in PHP.
Use the __toString()
Magic Method to Convert an Object to a String in PHP
We can use the __toString()
PHP magic method to convert an object to a string in PHP. There are some methods in PHP which start with __
, and PHP reserves them. When we perform some specific actions on objects, these methods override the default behavior. Some of the magic functions are __construct()
, __destruct()
, __isset()
, __set()
, __get()
, etc. We can use the __toString()
method to emulate an object to a string. Then, we can study the behavior of the object that acts like a string. Before PHP 8.0, the __toString()
method should always return a string value; otherwise, an error will be thrown. However, PHP 8.0 will coerce the value into a string if possible.
For example, create a class Student
and write a variable $name
as property and give it a name. Then, write the __toString()
function and return the $name
variable using the $this
keyword. Outside the class, create an object of the class Student
named $st
. Now, use the echo
function to print the $st
object.
In the output section, we can see the name is displayed. Hence, it proves that the object has been converted into a string. The echo
function is a string function, and we printed the string using it. This is how we can convert an object to a string using the magic method __toString()
.
Example Code:
<?php
class Student
{
protected $name = 'Jadon';
public function __toString()
{
return $this->name;
}
}
$st = new Student;
echo $st;
Output:
Jadon
Use the serialize()
Function to Convert an Object to a String in PHP
The serialize()
function in PHP converts the given value into a representation of a byte-stream string. We can use the function when we want to store the data in a session or database. The objects can be converted into a string using the serialize()
function. The function returns a sequence of bits. When we need to transfer bits across a network, the function comes into use.
For example, create the same class and member variable as in the first method. Then, create a function show()
. Using the echo
function inside the function to print the $name
variable using the $this
keyword. Outside the function, create an object $st
of the Student
class. Call the show()
function with the $st
object. Then use the serialize()
function on the object $st
and print it out with echo
function.
The example below outputs a string in a sequence of bits. Thus, we can convert a PHP object into a string using the serialize()
function.
Example Code:
<?php
class Student
{
protected $name = 'Jadon';
public function show()
{
echo $this->name."<br>";
}
}
$st = new Student;
$st->show();
$sr = serialize($st);
echo $sr;
Output:
Jadon
O:7:"Student":1:{s:7:"*name";s:5:"Jadon";}
Use the print_r()
Function to Convert an Object to a Strig in PHP
We can also use the print_r()
function to convert an object to a string in PHP. The function takes the first parameter as the value to be printed and the second parameter as the return parameter, a boolean value. We can supply an object as the first parameter and a true
boolean value as the second parameter to return a string. The return parameter is optional. The print_r()
function will print the value of the variable supplied if the second parameter is not supplied.
For example, create a class Student
with two member variables, $name
and $age
. Create a constructor with $name
and $age
as parameters and set the variables. Outside the class, create an object $st
and give the values jadon
and 21
as the arguments to the constructor. Then, create another variable $result
and assign print_r()
function to it. Set the function’s first parameter as $st
and true
as the second parameter. Finally, use the echo
function to print out the $result
.
When we set the return parameter of the print_r()
function to true
, then the function returned the string and stored it in the $result
variable. Thus, we could print the string using the echo
function. In this way, we can use the print_r()
function to convert the object into a string in PHP.
Example Code:
<?php
class Student
{
protected $name;
protected $age;
public function __construct($name, $age)
{
$this->name =$name;
$this->age = $age;
}
}
$st = new Student("jadon", 21);
$result = print_r($st, true);
echo $result;
Output:
Student Object ( [name:protected] => jadon [age:protected] => 21 )
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedInRelated Article - PHP Object
- Object Operator in PHP
- How to Define an Empty Object in PHP
- How to Create Default Object From Empty Value in PHP
- How to Create Array of Objects in PHP
- How to Create an Object Without Class in PHP