How to Convert an Array Into a CSV File in PHP
-
Use
fputcsv()
to Convert an Array Into a CSV File in PHP -
Use
fputcsv()
to Convert an Array Into a CSV File and Read That CSV File in PHP
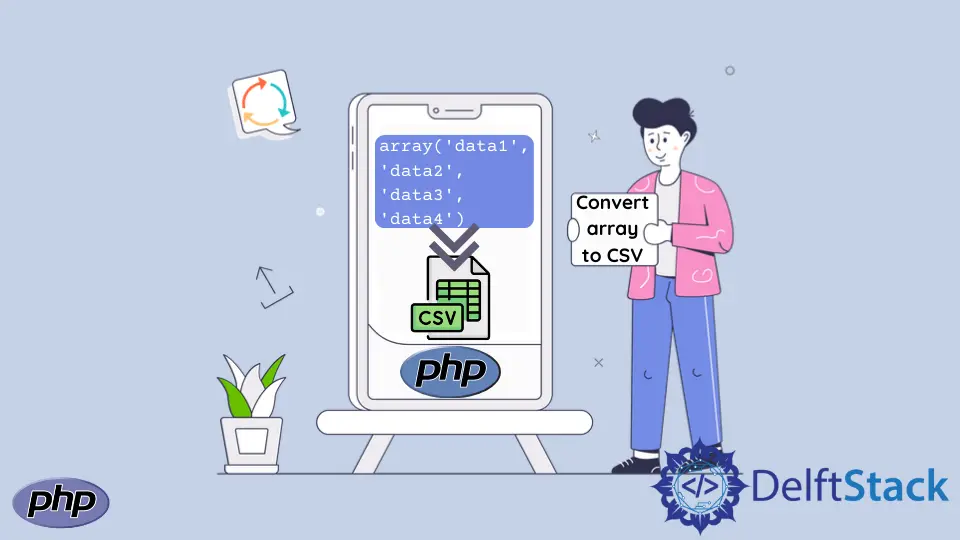
This tutorial will demonstrate using the fputcsv()
function to convert an array into a CSV file in PHP.
Use fputcsv()
to Convert an Array Into a CSV File in PHP
The fputcsv()
first formats a line as comma-separated values and then writes it to a CSV file. It takes a few parameters, two of which are required, the CSV file and the array of values.
fputcsv()
will put a single dimensional array as a row in the CSV file. We can use the foreach
loop for multidimensional arrays to put all the data into the CSV file.
It returns a string of values on success; otherwise, it returns False
.
Below is an example of code using fputcsv()
.
<?php
$data = array (
array('Delftstack1', 'Delftstack2', 'Delftstack3', 'Delftstack4'),
array('Delftstack1', 'Delftstack2', 'Delftstack3', 'Delftstack4'),
array('Delftstack1', 'Delftstack2', 'Delftstack3', 'Delftstack4'),
array('Delftstack1', 'Delftstack2', 'Delftstack3', 'Delftstack4')
);
//Create a CSV file
$file = fopen('Newfile.csv', 'w');
foreach ($data as $line) {
//put data into csv file
fputcsv($file, $line);
}
fclose($file);
?>
Output:
The above code first created a CSV file and wrote the data array one by one.
Use fputcsv()
to Convert an Array Into a CSV File and Read That CSV File in PHP
The code below first converts the given array to CSV using fputcsv()
with two more parameters, $delimiter
and $enclosure
. It reads the file and prints the string with all the file data.
See the example to understand better the fputcsv()
function with more parameters.
<?php
$data = array(
array('Employee', 'Salary', 'Attendence', 'Company'),
array('Mark', '3000', '20','Delftstack'),
array('Shawn', '4000', '22','Delftstack'),
array('Mike', '3500', '21','Delftstack')
);
$delimiter = ','; //parameter for fputcsv
$enclosure = '"'; //parameter for fputcsv
//convert array to csv
$file = fopen('file.csv', 'w+');
foreach ($data as $data_line) {
fputcsv($file, $data_line, $delimiter, $enclosure);
}
$data_read="";
rewind($file);
//read CSV
while (!feof($file)) {
$data_read .= fread($file, 8192); // will return a string of all data separeted by commas.
}
fclose($file);
echo $data_read;
?>
Output 1:
Employee,Salary,Attendence,Company
Mark,3000,20,Delftstack
Shawn,4000,22,Delftstack
Mike,3500,21,Delftstack
Output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook