How to Check if a Property Exists in PHP
-
Use
property_exists()
to Check if a Property Exists in PHP -
Use
isset()
to Check if a Property Exists in PHP - Conclusion
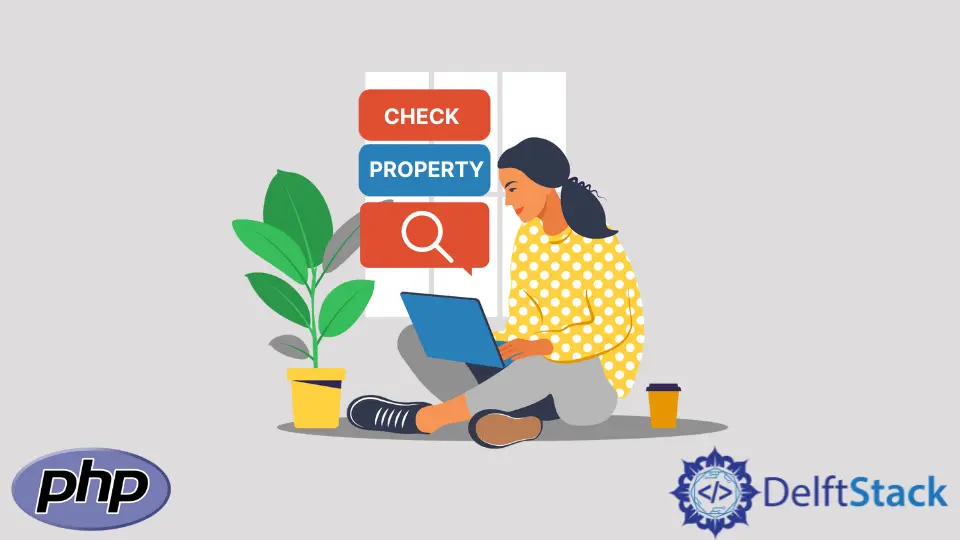
PHP gives us two straightforward solutions that we can use to check if a specific property exists in an object and then execute a particular piece of code.
This article will introduce these two ways to check if a property exists in an object or class in PHP.
Use property_exists()
to Check if a Property Exists in PHP
The property_exists()
method checks if the object or class has a property or not. It takes two arguments, the object and property.
Let’s go through an example in which we will check if a particular property exists or not. So let’s create an object with two properties, as shown below.
# php
$obj = (object) array('a' => 1, 'b' => 2);
Now, let’s check if the property c
exists in this object or not, as shown below.
# php
$obj = (object) array('a' => 1, 'b' => 2);
if(property_exists($obj, 'c')){
echo "Property C Exists";
}
if(property_exists($obj, 'b')){
echo "Property B Exists";
}
Output:
The property b
exists from the above example, and we get the above result.
Use isset()
to Check if a Property Exists in PHP
The isset()
function in PHP checks if a variable is set or if a variable exists or not. We can use the isset()
function to check if the object has a particular property declared or not.
This function is slightly different from property_exists()
because it returns true or false.
Let’s implement the isset()
function with the same scenario we implemented property_exists()
.
# php
if(isset($obj->c)){
echo "Property C Exists";
}
if(isset($obj->b)){
echo "Property B Exists";
}
Output:
As you can see, it returned the same result as the property_exists()
function.
Conclusion
So, in this tutorial, we learned about the two built-in functions in PHP that can be used to check if an object property exists or not and execute a certain piece of code if it does. We also learned the difference between isset()
and property_exists()
function.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn