How to Convert Celcius Degrees to Farenheit Using PHP
- Understanding the Formula for Conversion
- Method 1: Basic Conversion Function
- Method 2: Handling User Input
- Method 3: Converting an Array of Celsius Values
- Conclusion
- FAQ
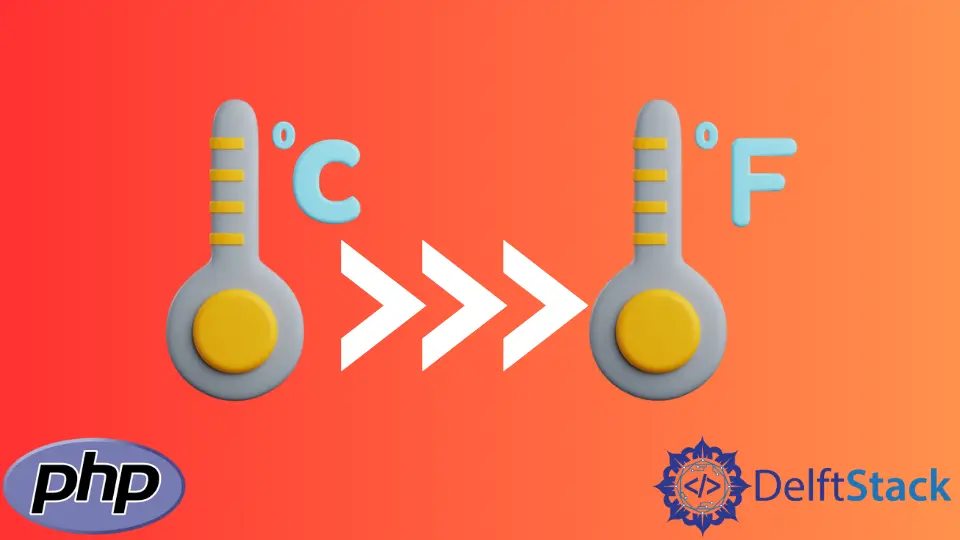
Converting Celsius to Fahrenheit is a common task in software development, especially when dealing with temperature data. Whether you’re building a weather application or simply need to display temperatures in a user-friendly format, knowing how to perform this conversion in PHP can be incredibly useful.
In this article, we will explore the straightforward method to convert Celsius degrees to Fahrenheit using PHP. We will provide clear code examples and detailed explanations to help you understand the conversion process. By the end of this guide, you’ll be equipped with the knowledge to implement this functionality in your own projects.
Understanding the Formula for Conversion
Before diving into the code, it’s essential to understand the formula used to convert Celsius to Fahrenheit. The formula is as follows:
F = (C × 9/5) + 32
Where:
- F is the temperature in Fahrenheit
- C is the temperature in Celsius
This formula is the backbone of our conversion process in PHP. Let’s see how to implement this in a simple PHP script.
Method 1: Basic Conversion Function
To create a basic conversion function in PHP, you can follow this simple code snippet:
<?php
function celsiusToFahrenheit($celsius) {
return ($celsius * 9/5) + 32;
}
$celsiusTemperature = 25; // Example temperature in Celsius
$fahrenheitTemperature = celsiusToFahrenheit($celsiusTemperature);
echo "Temperature in Fahrenheit: " . $fahrenheitTemperature;
?>
Output:
Temperature in Fahrenheit: 77
This code snippet defines a function named celsiusToFahrenheit
, which takes a Celsius temperature as an argument. It applies the conversion formula and returns the corresponding Fahrenheit value. In the example, we convert 25 degrees Celsius, which results in 77 degrees Fahrenheit.
This method is straightforward and efficient for converting temperatures. You can easily modify the $celsiusTemperature
variable to convert different Celsius values as needed.
Method 2: Handling User Input
In many applications, you may want to handle user input to perform the conversion dynamically. Here’s how you can create a simple PHP script that accepts user input:
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$celsiusTemperature = $_POST['celsius'];
$fahrenheitTemperature = celsiusToFahrenheit($celsiusTemperature);
echo "Temperature in Fahrenheit: " . $fahrenheitTemperature;
}
function celsiusToFahrenheit($celsius) {
return ($celsius * 9/5) + 32;
}
?>
<form method="post">
<label for="celsius">Enter Temperature in Celsius:</label>
<input type="number" name="celsius" required>
<input type="submit" value="Convert">
</form>
Output:
Temperature in Fahrenheit: 68
In this example, we use an HTML form to accept user input for the Celsius temperature. When the form is submitted, the script checks if the request method is POST and retrieves the Celsius value from the input field. It then calls the celsiusToFahrenheit
function to perform the conversion and displays the result.
This method allows users to input any temperature they wish to convert, making your application more interactive. You can further enhance this script by adding error handling or additional features, such as converting Fahrenheit back to Celsius.
Method 3: Converting an Array of Celsius Values
If you need to convert multiple Celsius values at once, you can modify your PHP script to handle arrays. Here’s a sample implementation:
<?php
function celsiusToFahrenheit($celsius) {
return ($celsius * 9/5) + 32;
}
$celsiusTemperatures = [0, 20, 37, 100]; // Example array of Celsius temperatures
$fahrenheitTemperatures = [];
foreach ($celsiusTemperatures as $celsius) {
$fahrenheitTemperatures[] = celsiusToFahrenheit($celsius);
}
foreach ($fahrenheitTemperatures as $fahrenheit) {
echo "Temperature in Fahrenheit: " . $fahrenheit . "\n";
}
?>
Output:
Temperature in Fahrenheit: 32
Temperature in Fahrenheit: 68
Temperature in Fahrenheit: 98.6
Temperature in Fahrenheit: 212
In this script, we define an array of Celsius temperatures. We then loop through each temperature, converting it to Fahrenheit using the celsiusToFahrenheit
function. The results are stored in a new array, which we then loop through to display each converted temperature.
This method is particularly useful when dealing with bulk data, such as when processing temperature readings from multiple sensors or datasets. It keeps your code organized and efficient while allowing for easy scalability.
Conclusion
Converting Celsius degrees to Fahrenheit in PHP is a simple yet essential task for any developer dealing with temperature data. Through the methods outlined in this article, you can easily implement temperature conversion in your applications, whether for user input, bulk processing, or basic conversions. By leveraging the power of PHP, you can create dynamic and user-friendly applications that cater to a wide range of temperature-related needs. Now that you have the tools and knowledge, feel free to experiment with these methods and enhance your PHP skills further.
FAQ
- How does the Celsius to Fahrenheit conversion formula work?
The formula F = (C × 9/5) + 32 converts Celsius to Fahrenheit by scaling the Celsius value and adjusting it to the Fahrenheit scale.
-
Can I convert Fahrenheit to Celsius using PHP?
Yes, you can use the formula C = (F - 32) × 5/9 to convert Fahrenheit back to Celsius. -
Is it possible to convert multiple Celsius values at once in PHP?
Absolutely! You can use arrays and loops to convert multiple Celsius values in a single script. -
What if the user inputs a non-numeric value?
You should implement error handling to validate user input and ensure that it is numeric before attempting the conversion. -
Can I use this conversion method in a web application?
Yes, this method can easily be integrated into any web application using PHP to handle temperature conversions dynamically.