Vector Addition in NumPy
- What is NumPy?
- Setting Up NumPy
- Method 1: Using the np.add() Function
- Method 2: Using the + Operator
- Method 3: Using np.sum() for Multi-dimensional Arrays
- Conclusion
- FAQ
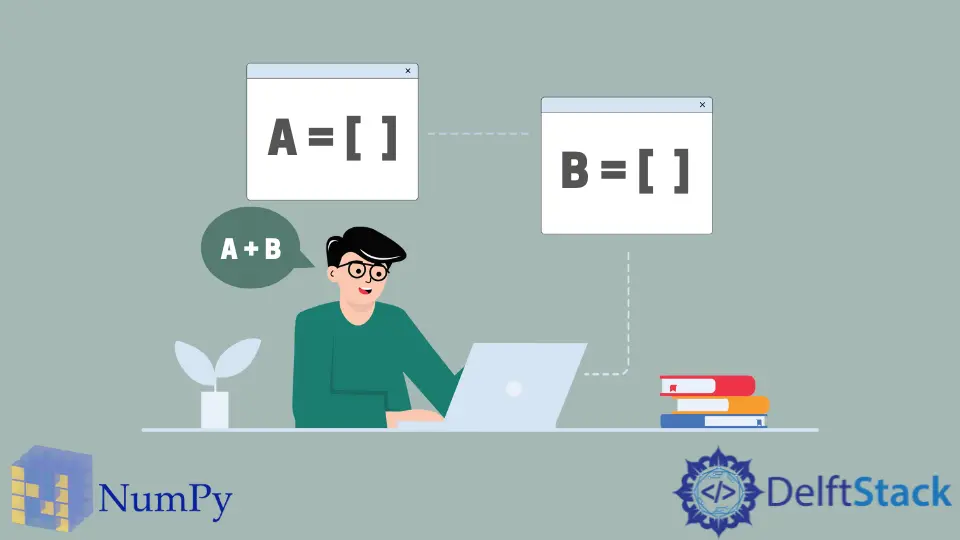
Vector addition is a fundamental operation in mathematics and computer science, particularly in fields like data analysis and machine learning. When working with numerical data in Python, NumPy is the go-to library for efficient array operations.
In this tutorial, we will explore how to perform vector addition using NumPy, showcasing its simplicity and power. Whether you’re a beginner or an experienced programmer, you’ll find valuable insights here. By the end of this article, you’ll be equipped with the knowledge to add vectors seamlessly, enhancing your data manipulation skills. Let’s dive into NumPy and unlock the potential of vector addition!
What is NumPy?
NumPy, short for Numerical Python, is a powerful library that provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. It is a cornerstone of scientific computing in Python and is widely used for data analysis, machine learning, and numerical simulations.
One of NumPy’s key features is its ability to perform vectorized operations, which means you can perform operations on entire arrays without the need for explicit loops. This not only makes your code cleaner but also significantly speeds up execution times, especially with large datasets. Vector addition is one of the simplest yet most essential operations you can perform using NumPy.
Setting Up NumPy
Before we start with vector addition, we need to ensure that we have NumPy installed. If you haven’t installed it yet, you can easily do so using pip. Open your terminal or command prompt and run the following command:
pip install numpy
Once installed, you can import NumPy into your Python script or Jupyter Notebook with the following line of code:
import numpy as np
Now that we have NumPy ready, let’s explore how to perform vector addition.
Method 1: Using the np.add() Function
The first method to add vectors in NumPy is by using the np.add()
function. This function takes two arrays as input and returns their element-wise sum. It’s straightforward and efficient, making it an excellent choice for beginners.
Here’s how you can use np.add()
to add two vectors:
import numpy as np
vector_a = np.array([1, 2, 3])
vector_b = np.array([4, 5, 6])
result = np.add(vector_a, vector_b)
print(result)
Output:
[5 7 9]
In this example, we first import the NumPy library and create two vectors, vector_a
and vector_b
, using np.array()
. The np.add()
function is then called with these two vectors as arguments. The result is stored in the variable result
, which contains the element-wise sum of the two vectors. As you can see, the output is a new vector where each element is the sum of the corresponding elements from the input vectors.
Using np.add()
is particularly useful when you want to maintain the original vectors unchanged, as it returns a new array without modifying the input arrays.
Method 2: Using the + Operator
Another simple way to perform vector addition in NumPy is by using the +
operator. This method is not only intuitive but also aligns closely with standard mathematical notation, making it easy to read and understand.
Here’s an example of adding two vectors using the +
operator:
import numpy as np
vector_a = np.array([7, 8, 9])
vector_b = np.array([1, 2, 3])
result = vector_a + vector_b
print(result)
Output:
[ 8 10 12]
In this code, we create two vectors, vector_a
and vector_b
, just like before. Instead of using a function, we simply use the +
operator to add them together. The result is stored in the variable result
, which again contains the element-wise sum. The output shows that each element from vector_a
has been added to the corresponding element from vector_b
.
Using the +
operator is often preferred for its simplicity and clarity, especially for those who are familiar with mathematical operations.
Method 3: Using np.sum() for Multi-dimensional Arrays
When dealing with multi-dimensional arrays, you might want to perform vector addition along a specific axis. The np.sum()
function can be particularly useful in this context. It allows you to specify the axis along which you want to perform the addition, making it flexible for various applications.
Let’s see how to use np.sum()
for vector addition in a multi-dimensional array:
import numpy as np
matrix_a = np.array([[1, 2], [3, 4]])
matrix_b = np.array([[5, 6], [7, 8]])
result = np.sum([matrix_a, matrix_b], axis=0)
print(result)
Output:
[[ 6 8]
[10 12]]
In this example, we create two 2D matrices, matrix_a
and matrix_b
. To add these matrices together, we use np.sum()
and pass a list containing both matrices. By specifying axis=0
, we indicate that we want to sum along the rows, effectively adding the corresponding elements from both matrices. The output shows a new matrix where each element is the sum of the corresponding elements from matrix_a
and matrix_b
.
This method is particularly useful when working with large datasets or when you want to aggregate data from multiple sources.
Conclusion
In this tutorial, we’ve explored three effective methods for performing vector addition using NumPy: the np.add()
function, the +
operator, and the np.sum()
function for multi-dimensional arrays. Each method has its advantages, and the choice depends on your specific use case and coding style. By mastering these techniques, you can enhance your data manipulation capabilities in Python, making your programming tasks more efficient and enjoyable. So, whether you’re working on a simple project or a complex data analysis task, NumPy’s vector addition features will undoubtedly come in handy.
FAQ
-
What is NumPy used for?
NumPy is used for numerical computing in Python, providing support for arrays, matrices, and a variety of mathematical functions. -
Can I perform vector addition with different-sized vectors in NumPy?
No, in NumPy, vectors must be of the same size to perform element-wise addition. -
Is NumPy faster than regular Python lists for mathematical operations?
Yes, NumPy is significantly faster than Python lists for mathematical operations due to its optimized performance and support for vectorized operations. -
How do I install NumPy?
You can install NumPy using pip by running the commandpip install numpy
in your terminal or command prompt.
- Can I add more than two vectors in NumPy?
Yes, you can add multiple vectors usingnp.add()
or the+
operator by chaining them together or using functions likenp.sum()
.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn