How to Shape and Size of Array in Python
- Understanding Array Shape in Python
- Finding Array Size in Python
- Reshaping Arrays in Python
- Conclusion
- FAQ
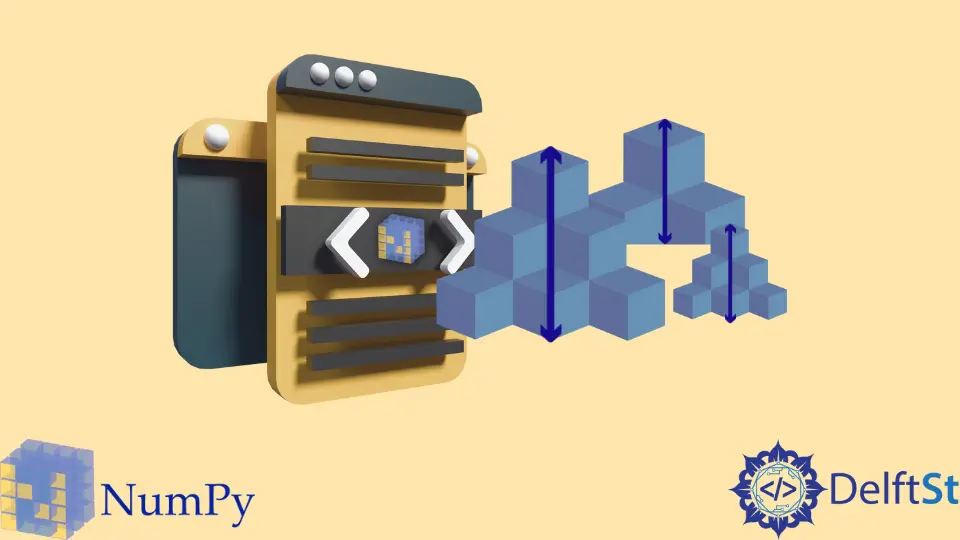
Understanding how to work with arrays is crucial for anyone diving into data science or numerical computing in Python. One of the most powerful libraries for handling arrays is NumPy.
In this article, we will explore the shape()
and size()
functions of the NumPy package, which are essential for determining the structure and size of arrays. Whether you’re a beginner or an experienced coder, mastering these functions will enhance your ability to manipulate data effectively. We will provide clear examples and explanations to ensure you grasp these concepts fully. So, let’s get started!
Understanding Array Shape in Python
The shape of an array in Python refers to the dimensions of the array, which indicate how many elements are present along each axis. For instance, a 2D array has rows and columns, while a 1D array has a single dimension. The shape
attribute of a NumPy array provides this information in the form of a tuple.
Here’s how you can find the shape of an array using NumPy:
import numpy as np
array_1d = np.array([1, 2, 3, 4, 5])
array_2d = np.array([[1, 2, 3], [4, 5, 6]])
shape_1d = array_1d.shape
shape_2d = array_2d.shape
print("Shape of 1D array:", shape_1d)
print("Shape of 2D array:", shape_2d)
Output:
Shape of 1D array: (5,)
Shape of 2D array: (2, 3)
In the example above, we created a 1D array with five elements and a 2D array with two rows and three columns. The shape
attribute returns a tuple that represents the dimensions of the array. For the 1D array, the shape is (5,)
, indicating that it has five elements in a single dimension. The 2D array’s shape is (2, 3)
, showing that it contains two rows and three columns. Understanding the shape of your arrays is fundamental for effective data manipulation.
Finding Array Size in Python
While the shape tells you the dimensions of your array, the size gives you the total number of elements present in that array. The size
attribute of a NumPy array provides this information, which is particularly useful when you need to know how much data you are working with.
Let’s see how to find the size of an array:
import numpy as np
array_1d = np.array([1, 2, 3, 4, 5])
array_2d = np.array([[1, 2, 3], [4, 5, 6]])
size_1d = array_1d.size
size_2d = array_2d.size
print("Size of 1D array:", size_1d)
print("Size of 2D array:", size_2d)
Output:
Size of 1D array: 5
Size of 2D array: 6
In this example, we again created a 1D and a 2D array. The size
attribute returns the total number of elements in each array. For the 1D array, the size is 5
, indicating there are five elements. For the 2D array, the size is 6
, which is the product of its shape dimensions (2 rows multiplied by 3 columns). Knowing the size of an array is crucial when performing operations like reshaping or when you’re processing large data sets.
Reshaping Arrays in Python
Sometimes, you may need to change the shape of an array without changing its data. The reshape()
function in NumPy allows you to do just that. It’s important to ensure that the new shape is compatible with the total number of elements in the original array.
Here’s how you can reshape an array:
import numpy as np
array_1d = np.array([1, 2, 3, 4, 5, 6])
reshaped_array = array_1d.reshape((2, 3))
print("Original 1D array:", array_1d)
print("Reshaped 2D array:\n", reshaped_array)
Output:
Original 1D array: [1 2 3 4 5 6]
Reshaped 2D array:
[[1 2 3]
[4 5 6]]
In this code snippet, we created a 1D array with six elements and then reshaped it into a 2D array with two rows and three columns. The reshape()
function takes a tuple that specifies the new shape. It’s essential to ensure that the total number of elements remains the same; otherwise, NumPy will raise an error. Reshaping is particularly useful when preparing data for machine learning models or when you need to visualize data in a specific format.
Conclusion
In this article, we’ve explored how to determine the shape and size of arrays in Python using the NumPy library. Understanding the shape()
and size()
functions is crucial for effective data manipulation and analysis. We also looked at how to reshape arrays to fit specific requirements. Mastering these concepts will significantly enhance your ability to work with data in Python, paving the way for more advanced techniques in data science and machine learning. Keep practicing, and soon you’ll find yourself manipulating arrays like a pro!
FAQ
-
What is the difference between shape and size in NumPy?
Shape refers to the dimensions of the array, while size indicates the total number of elements in the array. -
Can I reshape an array to an incompatible size?
No, reshaping an array must maintain the same total number of elements; otherwise, NumPy will raise an error. -
How do I create a multi-dimensional array in NumPy?
You can create multi-dimensional arrays using nested lists or tuples when initializing the array withnp.array()
. -
What happens if I try to access an index outside the array’s shape?
Attempting to access an index outside the array’s shape will result in an IndexError. -
Can I change the shape of an array in place?
Yes, you can use theresize()
method to change the shape of an array in place, but it may alter the data if the new shape does not match the original size.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn