How to Read CSV to NumPy Array in Python
-
Use the
numpy.genfromtxt()
Function to Read CSV Data to a NumPy Array -
Use a
pandas
DataFrame to Read CSV Data to a NumPy Array -
Use the
csv
Module to Read CSV Data to a NumPy Array
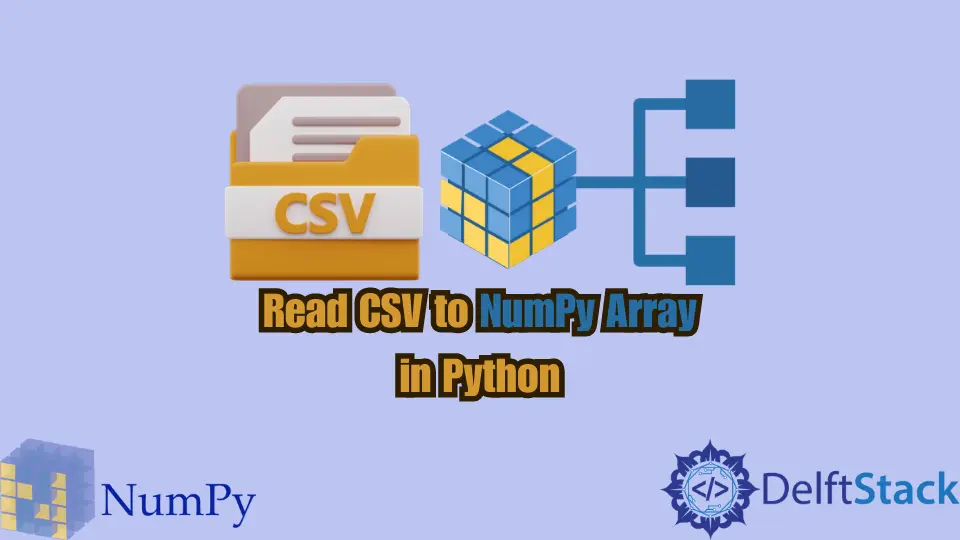
This tutorial will discuss how to read data from a CSV file and store it in a numpy array.
Use the numpy.genfromtxt()
Function to Read CSV Data to a NumPy Array
The genfromtxt()
function is frequently used to load data from text files. We can read data from CSV files using this function and store it in a numpy array. This function has many arguments available, making it easier to load the data in our desired format. We can specify the delimiter, deal with missing values, delete specified characters, and specify the datatype of the data using the different arguments of this function.
For example,
from numpy import genfromtxt
data = genfromtxt("sample.csv", delimiter=",", skip_header=1)
print(data)
Output:
[[1. 2. 3.]
[4. 5. 6.]]
Many other functions are derived from this, like recfromtxt()
and recfromcsv()
that can perform the same function but have different default values.
In the following code, we will read data from a CSV file using the recfromcsv()
file.
import numpy as np
data = np.recfromcsv("sample.csv", skip_header=0)
print(data)
Output:
[(1, 2, 3) (4, 5, 6)]
Note that we didn’t have to specify the delimiter as a comma and the different value to specify the header row.
Use a pandas
DataFrame to Read CSV Data to a NumPy Array
We can also make use of a pandas
DataFrame to read CSV data into an array. For this, we will read the data to a DataFrame and then convert this to a NumPy array using the values()
function from the pandas
library.
The following code implements this.
from pandas import read_csv
df = read_csv("sample.csv")
data = df.values
print(data)
Output:
[[1 2 3]
[4 5 6]]
Use the csv
Module to Read CSV Data to a NumPy Array
The csv
module is used to read and write data to CSV files efficiently. This method will read the data from a CSV file using this module and store it in a list. We will then proceed to convert this list to a numpy array.
The code below will explain this.
import csv
import numpy as np
with open("sample.csv", "r") as f:
data = list(csv.reader(f, delimiter=";"))
data = np.array(data)
print(data)
Output:
[['1,2,3']
['4,5,6']]
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn