How to Convert Pandas Series to NumPy Array
-
Convert Pandas Series to NumPy Array With the
pandas.index.values
Property -
Convert Pandas Series to NumPy Array With the
pandas.index.to_numpy()
Function -
Convert Pandas Series to NumPy Array With the
pandas.index.array
Property
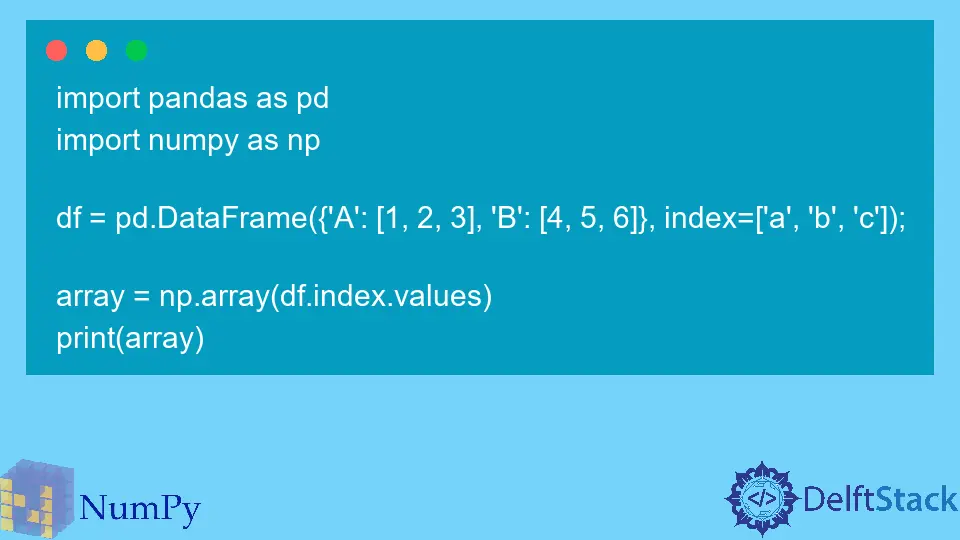
This tutorial will discuss how to convert the Pandas series to a NumPy array in Python.
Convert Pandas Series to NumPy Array With the pandas.index.values
Property
If we want to convert a Pandas series to a NumPy array, we can use the pandas.index.values
property. The pandas.index.values
property returns the values at the index in the form of an array. We can then convert this array into a NumPy array with the numpy.array()
function. See the following code example.
import pandas as pd
import numpy as np
df = pd.DataFrame({"A": [1, 2, 3], "B": [4, 5, 6]}, index=["a", "b", "c"])
array = np.array(df.index.values)
print(array)
Output:
['a' 'b' 'c']
We first created the Pandas series df
with the pd.DataFrame()
function. We then converted the df
to an array with the df.index.values
property and stored it inside the NumPy array array
with the np.array()
function.
Convert Pandas Series to NumPy Array With the pandas.index.to_numpy()
Function
The pandas.index.values
method works fine for now but is deprecated and will be removed in the future versions of the Pandas package. A good replacement for the pandas.index.values
property is the pandas.index.to_numpy()
function. The pandas.index.to_numpy()
function directly converts the values inside the Pandas series to a NumPy array, so we do not need to use the numpy.array()
function explicitly. The following code example shows us how to convert Pandas series to NumPy array with the pandas.index.to_numpy()
function.
import pandas as pd
df = pd.DataFrame({"A": [1, 2, 3], "B": [4, 5, 6]}, index=["a", "b", "c"])
array = df.index.to_numpy()
print(array)
Output:
['a' 'b' 'c']
We first created the Pandas series df
with the pd.DataFrame()
function. We then converted the df
to a NumPy array with the df.index.to_numpy()
function and stored the result inside the array
.
Convert Pandas Series to NumPy Array With the pandas.index.array
Property
Another method that can be used in place of the pandas.index.values
property is the pandas.index.array
property. The pandas.index.array
property converts the Pandas series to a Pandas array. We can convert this Pandas array to a NumPy array with the numpy.array()
function. See the following code example.
import pandas as pd
import numpy as np
df = pd.DataFrame({"A": [1, 2, 3], "B": [4, 5, 6]}, index=["a", "b", "c"])
array = np.array(df.index.array)
print(array)
Output:
['a' 'b' 'c']
We first created the Pandas series df
with the pd.DataFrame()
function. We then converted the df
to a Pandas array with the df.index.array
property and stored the result inside the NumPy array array
with the numpy.array()
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn