numpy.newaxis Method
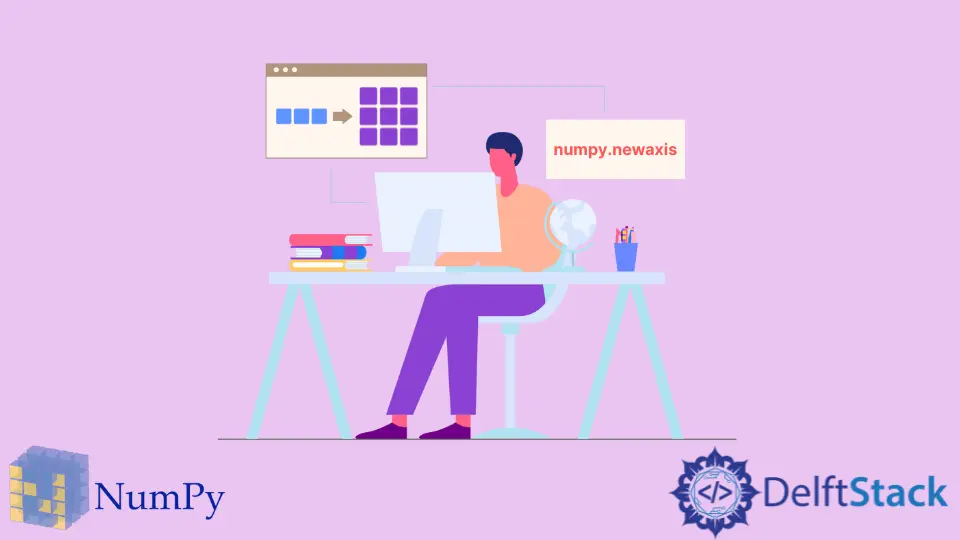
This tutorial will discuss the numpy.newaxis
method.
the numpy.newaxis
Method
The numpy.newaxis
method is an alias for the None
, which is used for array indexing in Python. The most straightforward use of the numpy.newaxis
is to add a new dimension to a NumPy array in Python. For example, converting a 1D array into a 2D array, converting a 2D array into a 3D array, and so on. We can also convert a 1D array to either a row matrix or a column matrix with the numpy.newaxis
in Python. The following code example shows us how to transform a 1D array into a row matrix with the numpy.newaxis
in Python.
import numpy as np
array = np.array([1, 2, 3, 4])
print(array.shape)
array = array[np.newaxis]
print(array.shape)
Output:
(4,)
(1, 4)
We transformed the 1D array array
into a row matrix with the np.newaxis
in the above code. We first created the 1D array array
with the np.array()
function. We then used the [np.newaxis]
as the index of the array
to return a row matrix. The following code example shows us how to transform a 1D array into a column matrix with the numpy.newaxis
.
import numpy as np
array = np.array([1, 2, 3, 4])
print(array.shape)
array = array[:, np.newaxis]
print(array.shape)
Output:
(4,)
(4, 1)
We transformed the 1D array array
into a column matrix with the np.newaxis
in the above code. We first created the 1D array array
with the np.array()
function. We then used the [:, np.newaxis]
as the index of the array
to return a column matrix.
As we have mentioned previously, the numpy.newaxis
is an alias for None
. So, we can also perform both operations discussed above with the None
in place of the numpy.newaxis
. The following code example shows us how to transform a 1D array into a row matrix with the None
in Python.
import numpy as np
array = np.array([1, 2, 3, 4])
print(array.shape)
array = array[None]
print(array.shape)
Output:
(4,)
(1, 4)
We transformed the 1D array array
into a row matrix with the None
in Python in the above code. We first created the 1D array array
with the np.array()
function. We then used the [None]
as the index of the array
to return a row matrix.
The following code example shows us how to transform a 1D array into a column matrix with the None
in Python.
import numpy as np
array = np.array([1, 2, 3, 4])
print(array.shape)
array = array[:, None]
print(array.shape)
Output:
(4,)
(4, 1)
We transformed the 1D array array
into a column matrix with the None
in Python in the above code. We first created the 1D array array
with the np.array()
function. We then used the [:, None]
as the index of the array
to return a column matrix.
In conclusion, both numpy.newaxis
, and None
are the same and can be used in array indexing and slicing.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn