How to Count Unique Values in NumPy Array
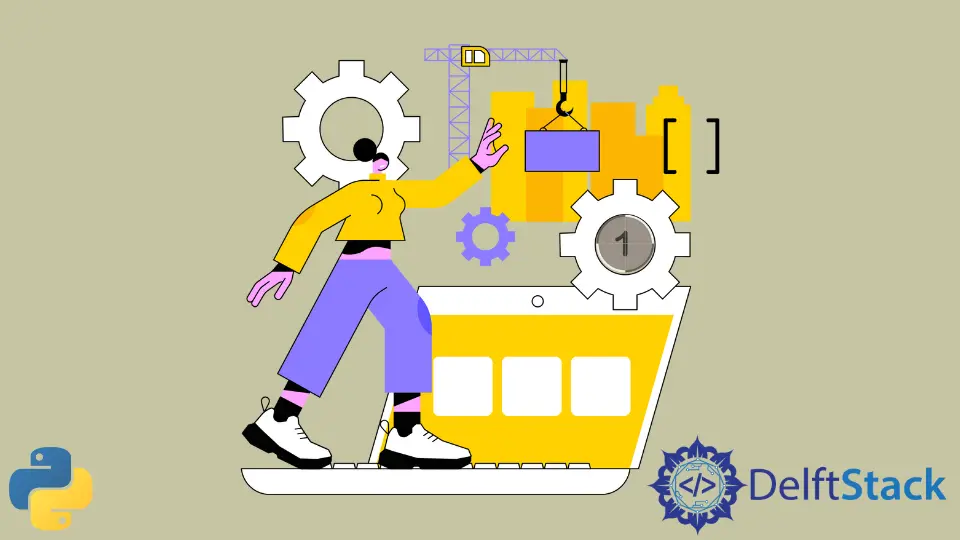
This tutorial will introduce how to count unique values’ occurrences inside a NumPy array.
Count Unique Values in NumPy Array With the numpy.unique()
Function
To count each unique element’s number of occurrences in the numpy array, we can use the numpy.unique()
function. It takes the array as an input argument and returns all the unique elements inside the array in ascending order. We can specify the return_counts
parameter as True
to also get the number of times each element is repeated inside the array. See the following code example.
import numpy as np
array = np.array([1, 1, 1, 2, 3, 4, 4, 4])
unique, counts = np.unique(array, return_counts=True)
result = np.column_stack((unique, counts))
print(result)
Output:
[[1 3]
[2 1]
[3 1]
[4 3]]
We first created a NumPy array with the np.array()
function. We then stored all the unique elements of the array
inside the unique
array and their respective number of occurrences inside the counts
array with the np.unique()
function. We then zipped the two 1D arrays unique
and counts
inside a single 2D array result
with the np.column_stack()
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn