NumPy Unit Vector
-
Get Unit Vector From NumPy Array With the
numpy.linalg.norm()
Function - Get Unit Vector From NumPy Array With the Self-Defined Approach
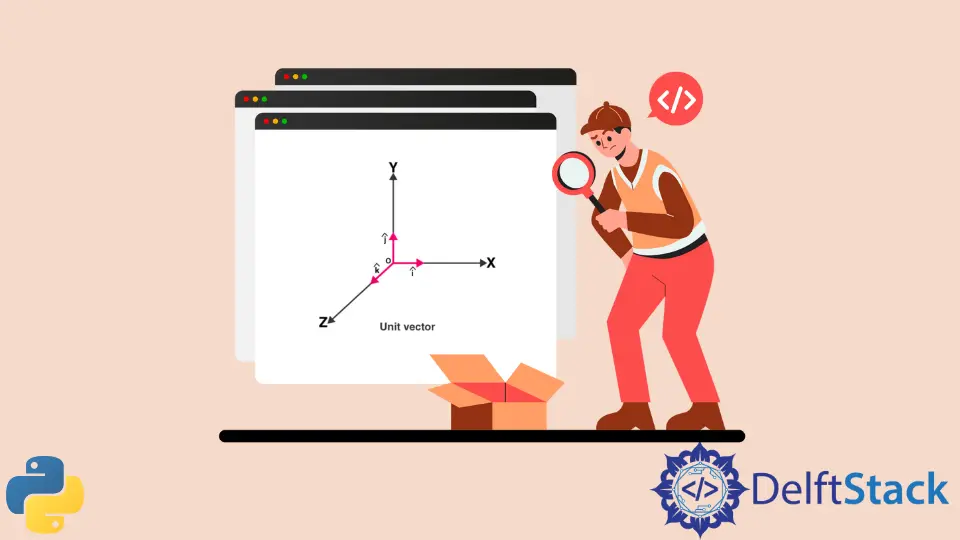
This tutorial will discuss the method to normalize a NumPy array to a unit vector in Python.
Get Unit Vector From NumPy Array With the numpy.linalg.norm()
Function
A vector is a quantity that has a magnitude as well as a direction. A unit vector is a vector whose magnitude is equal to one. We can normalize a vector to its corresponding unit vector with the help of the numpy.linalg.norm()
function. The numpy.linalg
library contains a lot of functions related to linear algebra. We can use the norm()
function inside the numpy.linalg
to calculate the norm of a vector. We can divide the vector by its norm to get the unit vector of the vector.
import numpy as np
vector = np.array([1, 2, 3])
unit_vector = vector / np.linalg.norm(vector)
print(unit_vector)
Output:
[0.26726124 0.53452248 0.80178373]
We first created the vector with the numpy.array()
function. We then calculated the unit vector of the vector by dividing the vector with the norm of the vector and saved the result inside the unit_vector
.
Get Unit Vector From NumPy Array With the Self-Defined Approach
We can also calculate the unit vector without using the norm()
function inside the numpy.linalg
library in Python. We can find the norm by calculating the square root of the sum of squares of each element inside the vector. We can then calculate the unit vector by dividing the vector by its norm. See the following code example.
import numpy as np
vector = np.array([1, 2, 3])
unit_vector = vector / (vector ** 2).sum() ** 0.5
print(unit_vector)
Output:
[0.26726124 0.53452248 0.80178373]
We first created the vector with the numpy.array()
function. We then calculated the unit vector of the vector by dividing the vector with the norm of the vector and saved the result inside the unit_vector
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn