How to Rank Values in NumPy Array
-
NumPy Rank With the
numpy.argsort()
Method -
NumPy Rank With
scipy.stats.rankdata()
Function in Python
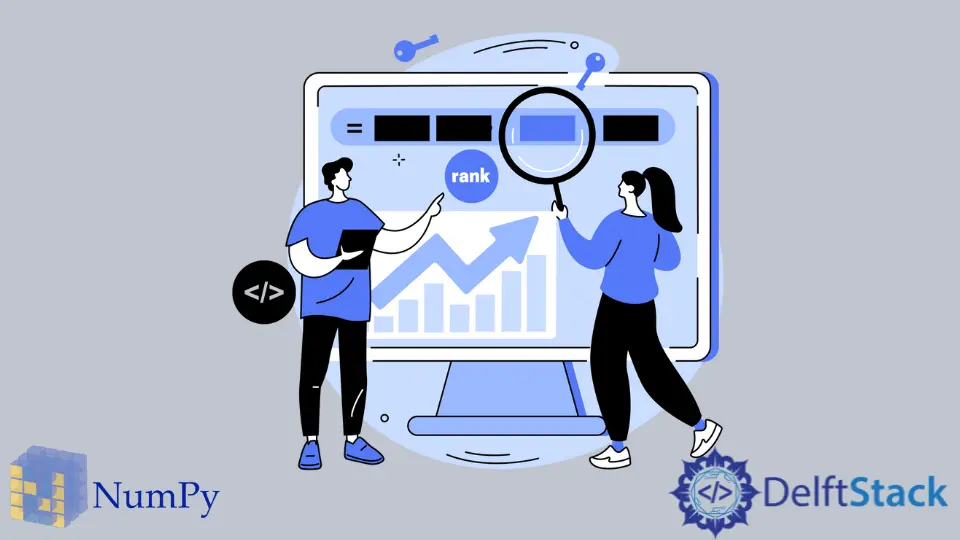
This tutorial will introduce the methods to rank data inside a Python NumPy array.
NumPy Rank With the numpy.argsort()
Method
The numpy.argsort()
method is used to get the indices that can be used to sort a NumPy array. These indices can also be used as ranks for each element inside the array. The numpy.argsort()
method is called by the array and returns the rank of each element inside the array in the form of another array.
import numpy as np
array = np.array([1, 8, 5, 7, 9])
temp = array.argsort()
ranks = np.empty_like(temp)
ranks[temp] = np.arange(len(array))
print(array)
print(ranks)
Output:
[1 8 5 7 9]
[0 3 1 2 4]
We ranked the elements inside the NumPy array array
using the numpy.argsort()
function in the above code. We first created our array with the np.array()
function. We then used the array.argsort()
function and stored the values inside the temp
array. After that, we created another array, ranks
, that contains the rank of each element in the array
. We then assigned the rank of each element inside the array
to each element of the ranks
with ranks[temp] = np.arange(len(array))
.
The method discussed in the above-mentioned coding example works fine, but we can further simplify our code using the numpy.argsort()
function twice. This phenomenon is demonstrated in the coding example below.
import numpy as np
array = np.array([1, 8, 5, 7, 9])
temp = array.argsort()
ranks = temp.argsort()
print(array)
print(ranks)
Output:
[1 8 5 7 9]
[0 3 1 2 4]
We created another array ranks
and assigned the rank of each element inside the array
to each element of the ranks
with ranks = temp.argsort()
.
NumPy Rank With scipy.stats.rankdata()
Function in Python
We can also use the rankdata()
function inside the scipy.stats
library to get the rank of each element inside our NumPy array. The rankdata()
function takes the array as an input parameter, ranks each element inside the array, and returns the result in the form of another array of the same length.
from scipy.stats import rankdata
import numpy as np
array = np.array([1, 8, 5, 7, 9])
ranks = rankdata(array)
print(array)
print(ranks)
Output:
[1 8 5 7 9]
[1. 4. 2. 3. 5.]
We first created our array with the np.array()
function. We then used the rankdata(array)
function and stored the values inside the ranks
array.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn