How to numpy.random.seed() Function in NumPy
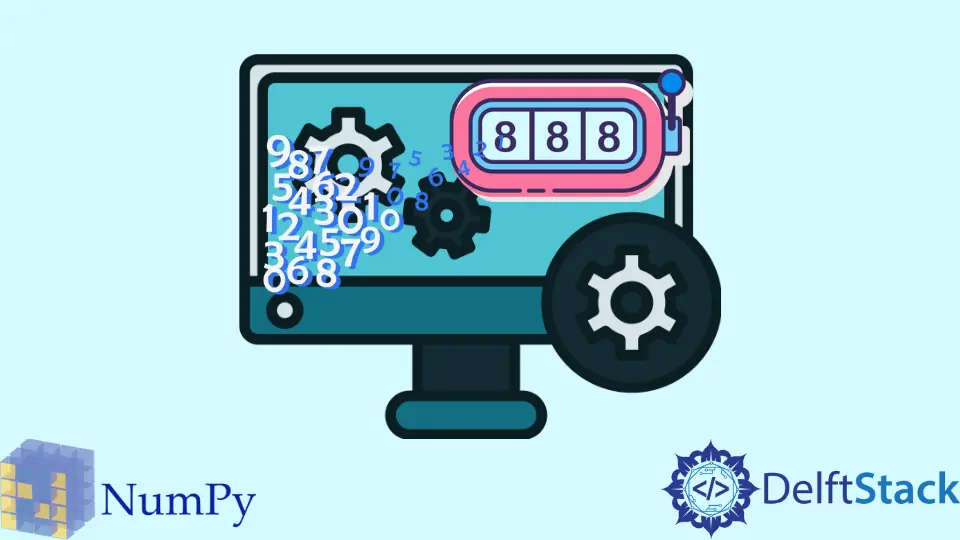
This tutorial will explain the numpy.random.seed()
function in NumPy.
numpy.random.seed()
Function
The numpy.random.seed()
function is used to set the seed for the pseudo-random number generator algorithm in Python. The pseudo-random number generator algorithm performs some predefined operations on the seed and produces a pseudo-random number in the output. The seed acts as a starting point for the algorithm. A pseudo-random number is a number that appears random, but it actually isn’t. In fact, computers are incapable of generating a truly random number because computers are deterministic and consistently follow a given set of instructions. The idea behind this is that we will always get the same set of random numbers for the same seed on any machine.
import numpy as np
np.random.seed(1)
array = np.random.rand(5)
np.random.seed(1)
array2 = np.random.rand(5)
print(array)
print(array2)
Output:
[4.17022005e-01 7.20324493e-01 1.14374817e-04 3.02332573e-01
1.46755891e-01]
[4.17022005e-01 7.20324493e-01 1.14374817e-04 3.02332573e-01
1.46755891e-01]
In the above code, we set the NumPy random seed to 0 and generated a sequence of five pseudo-random numbers based on that seed. We then reset the seed to 0 and again generated a sequence of five pseudo-random numbers based on that seed. Notice that both times we get the same sequence of values. Both times, the random numbers are generated by applying the same operations on the same seeds. This pre-deterministic random number generation type is helpful when we want to generate the same sequence of random numbers on different machines.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn