numpy.random.permutation() Function in NumPy
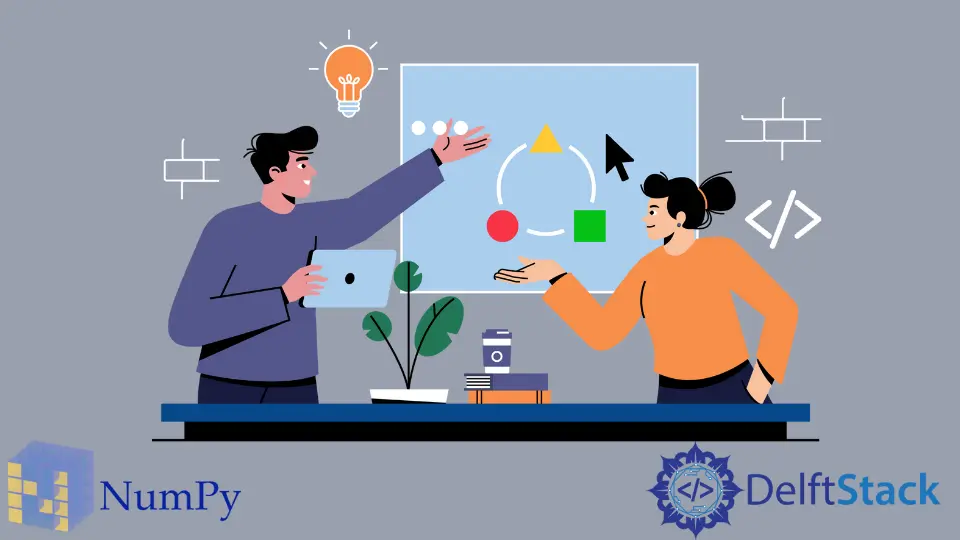
This tutorial will introduce the methods to upgrade the NumPy package in Python.
NumPy Random Permutation With the numpy.random.permutation()
Function in Python
The numpy.random.permutation()
function is mainly used for two purposes: to get a randomly permuted copy of a sequence and get a randomly permuted range in Python. The key differences between the permutation()
and shuffle()
functions are that if passed an array, the permutation()
function returns a shuffled copy of the original array. In contrast, the shuffle()
function shuffles the original array. And, if we pass an integer, the permutation()
function gives us a randomly permuted sequence of numbers with the given length, while to do that same process requires us to use the numpy.arange()
function with the shuffle()
function. The following code examples demonstrate the differences between the permutation()
function and the shuffle()
function in Python.
Example#1:
import numpy as np
array = np.array([0,1,0,0,4])
shuffled = np.random.permutation(array)
np.random.shuffle(array)
print(shuffled)
print(array)
Output:
[0 0 4 1 0]
[0 4 0 1 0]
Example#2:
permuted = np.random.permutation(5)
print(permuted)
sequence = np.arange(5)
np.random.shuffle(sequence)
print(sequence)
Output:
[3 1 4 0 2]
[4 3 0 1 2]
In the first example, we demonstrated the difference between the permutation()
function and the shuffle()
function when an array is passed to both functions. The permutation()
function returns a shuffled copy of the array
while the shuffle()
function shuffles the original array
.
In the second example, we demonstrated the difference between the permutation()
function and the shuffle()
function when an integer is passed. The permutation(n)
function returns a shuffled array with a sequence of n
integer elements, while to imitate this behavior with the shuffle()
function, we have first to create a sequence of n
integers with the np.arange()
function. The shuffle()
function then shuffles this newly created sequence of integer elements.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn