How to Print Full NumPy Array
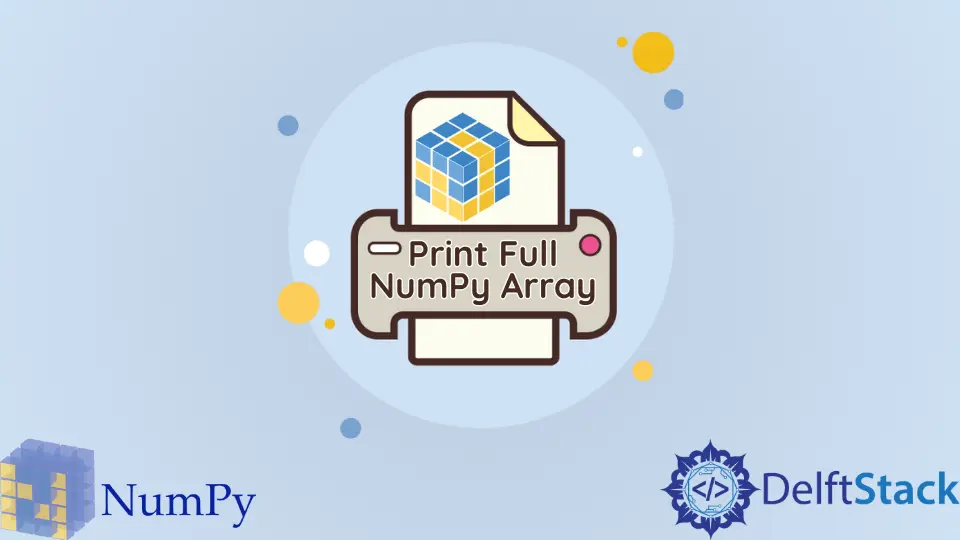
This tutorial will introduce how to print a full NumPy array in Python.
Print Full NumPy Array With the numpy.set_printoptions()
Function in Python
By default, if our array’s length is huge, Python will truncate the output when the array is printed. This phenomenon is demonstrated in the code example below.
import numpy as np
array = np.arange(10000)
print(array)
Output:
[ 0 1 2 ... 9997 9998 9999]
In the above code, we first created a NumPy array array
that contains numerical values from 0 to 9999 with the np.arange()
function in Python. We then printed the elements of the array with the print()
function. We get a truncated output because the array is too large to be displayed completely.
This problem can be solved by with the numpy.set_printoptions()
function. It sets different parameters related to the printing arrays in Python. We can use the threshold
parameter of the numpy.set_printoptions()
function to sys.maxsize
to print the complete NumPy array. To use the sys.maxsize
property, we have to import the sys
library as well. The following code example shows how to print a full NumPy array with the numpy.set_printoptions()
function and the sys.maxsize
property in Python.
import sys
import numpy as np
array = np.arange(10001)
np.set_printoptions(threshold=sys.maxsize)
print(array)
Output:
[ 0 1 2 3 4 5 6 7 8 9 10 11
12 13 14 15 16 17 18 19 20 21 22 23
24 25 26 27 28 29 30 31 32 33 34 35
36 37 38 39 40 41 42 43 44 45 46 47
48 49 50 51 52 53 54 55 56 57 58 59
60 61 62 63 64 65 66 67 68 69 70 71
72 73 74 75 76 77 78 79 80 81 82 83
84 85 86 87 88 89 90 91 92 93 94 95
96 97 98 99 100 101 102 103 104 105 106 107
108 109 110 111 112 113 114 115 116 117 118 119
...
9912 9913 9914 9915 9916 9917 9918 9919 9920 9921 9922 9923
9924 9925 9926 9927 9928 9929 9930 9931 9932 9933 9934 9935
9936 9937 9938 9939 9940 9941 9942 9943 9944 9945 9946 9947
9948 9949 9950 9951 9952 9953 9954 9955 9956 9957 9958 9959
9960 9961 9962 9963 9964 9965 9966 9967 9968 9969 9970 9971
9972 9973 9974 9975 9976 9977 9978 9979 9980 9981 9982 9983
9984 9985 9986 9987 9988 9989 9990 9991 9992 9993 9994 9995
9996 9997 9998 9999 10000]
In the above code, we first created a NumPy array array
that contains elements from 0 to 10000 with the numpy.arange()
function. We set the print options for the array to be maximum with the np.set_printoptions(threshold = sys.maxsize)
function. We then printed the full array with the simple print()
function in Python.
There is another solution to our problem that involves only the use of the NumPy
library. We can specify the threshold
inside the numpy.set_printoptions()
function to be equal to np.inf
to print the complete array in Python. The np.inf
property specifies that the print()
will run infinitely until the whole array is printed. See the following code example.
import numpy as np
array = np.arange(10001)
np.set_printoptions(threshold=np.inf)
print(array)
Output:
[ 0 1 2 3 4 5 6 7 8 9 10 11
12 13 14 15 16 17 18 19 20 21 22 23
24 25 26 27 28 29 30 31 32 33 34 35
36 37 38 39 40 41 42 43 44 45 46 47
48 49 50 51 52 53 54 55 56 57 58 59
60 61 62 63 64 65 66 67 68 69 70 71
72 73 74 75 76 77 78 79 80 81 82 83
84 85 86 87 88 89 90 91 92 93 94 95
96 97 98 99 100 101 102 103 104 105 106 107
108 109 110 111 112 113 114 115 116 117 118 119
...
9912 9913 9914 9915 9916 9917 9918 9919 9920 9921 9922 9923
9924 9925 9926 9927 9928 9929 9930 9931 9932 9933 9934 9935
9936 9937 9938 9939 9940 9941 9942 9943 9944 9945 9946 9947
9948 9949 9950 9951 9952 9953 9954 9955 9956 9957 9958 9959
9960 9961 9962 9963 9964 9965 9966 9967 9968 9969 9970 9971
9972 9973 9974 9975 9976 9977 9978 9979 9980 9981 9982 9983
9984 9985 9986 9987 9988 9989 9990 9991 9992 9993 9994 9995
9996 9997 9998 9999 10000]
We set the threshold
parameter to be np.inf
with the np.set_printoptions()
function. We then printed the full array with the simple print()
function in Python. This approach is preferred over the previous method because this approach only requires the NumPy
library.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn