How to Normalize Matrix in NumPy
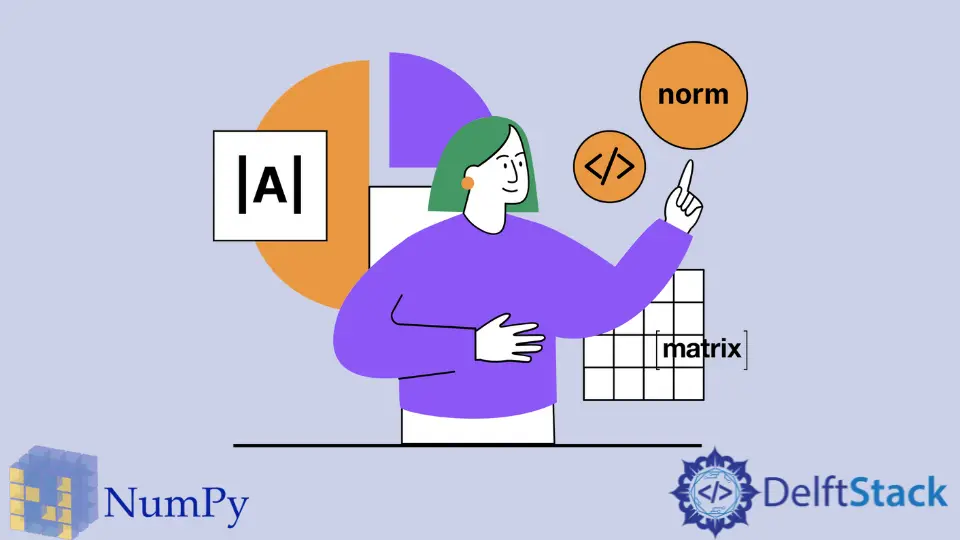
This tutorial will discuss the method to normalize a matrix in Python.
Normalize Matrix With the numpy.linalg.norm()
Method in Python
The numpy.linalg
library contains methods related to linear algebra in Python. The norm()
method inside the numpy.linalg
calculates the norm of a matrix. We can then use these norm values to normalize a matrix. The following code example shows us how we can normalize a matrix with the norm()
method inside the numpy.linalg
library.
import numpy as np
matrix = np.array([[1, 2], [3, 4]])
norms = np.linalg.norm(matrix, axis=1)
print(matrix / norms)
Output:
[[0.4472136 0.4 ]
[1.34164079 0.8 ]]
We first created our matrix in the form of a 2D array with the np.array()
method. We then calculated the norm and stored the results inside the norms
array with norms = np.linalg.norm(matrix)
. In the end, we normalized the matrix
by dividing it with the norms
and printed the results.
The norm()
method performs an operation equivalent to np.sqrt(1**2 + 2**2)
and np.sqrt(3**2 + 4**2)
on the first and second row of our matrix, respectively. It then allocates two values to our norms
array, which are [2.23606798 5.0]
. The matrix is then normalized by dividing each row of the matrix
by each element of norms
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn