NumPy Matrix Vector Multiplication
-
NumPy Matrix Vector Multiplication With the
numpy.matmul()
Method -
NumPy Matrix Vector Multiplication With the
numpy.dot()
Method
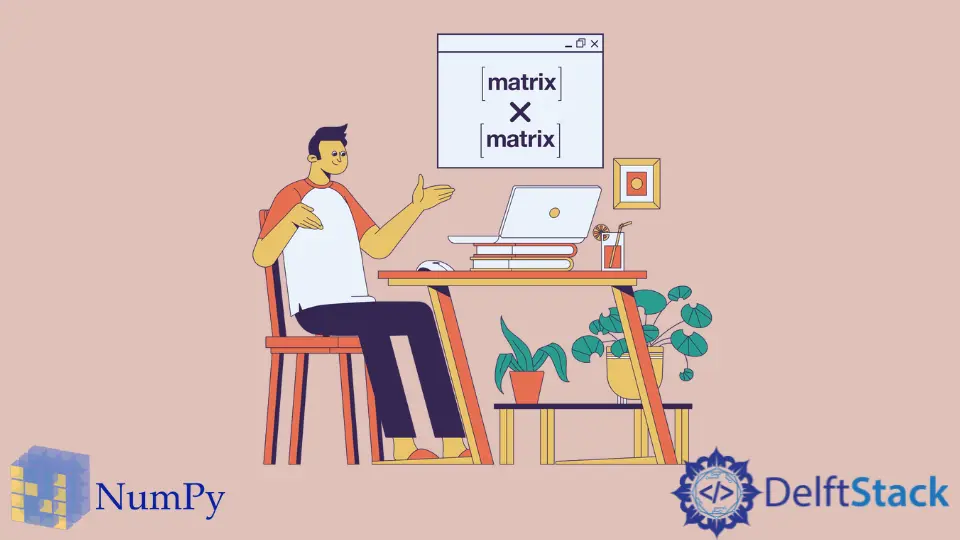
This tutorial will introduce the methods to multiply two matrices in NumPy.
NumPy Matrix Vector Multiplication With the numpy.matmul()
Method
To calculate the product of two matrices, the column number of the first matrix must be equal to the row number of the second matrix. The numpy.matmul()
method is used to calculate the product of two matrices. The numpy.matmul()
method takes the matrices as input parameters and returns the product in the form of another matrix. See the following code example.
import numpy as np
m1 = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
m2 = np.array([[9, 8, 7, 6], [5, 4, 3, 3], [2, 1, 2, 0]])
m3 = np.matmul(m1, m2)
print(m3)
Output:
[[ 25 19 19 12]
[ 73 58 55 39]
[121 97 91 66]]
We first created the matrices in the form of 2D arrays with the np.array()
method. We then calculated the product of both matrices with the np.matmul(m1,m2)
method and stored the result inside the m3
matrix.
NumPy Matrix Vector Multiplication With the numpy.dot()
Method
The numpy.dot()
method calculates the dot product of two arrays. It can also be used on 2D arrays to find the matrix product of those arrays. The numpy.dot()
method takes two matrices as input parameters and returns the product in the form of another matrix. See the following code example.
import numpy as np
m1 = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
m2 = np.array([[9, 8, 7, 6], [5, 4, 3, 3], [2, 1, 2, 0]])
m3 = np.dot(m1, m2)
print(m3)
Output:
[[ 25 19 19 12]
[ 73 58 55 39]
[121 97 91 66]]
We first created the matrices in the form of 2D arrays with the np.array()
method. We then calculated the product of both matrices with the np.dot(m1,m2)
method and stored the result inside the m3
matrix.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn