NumPy Matrix Subtraction
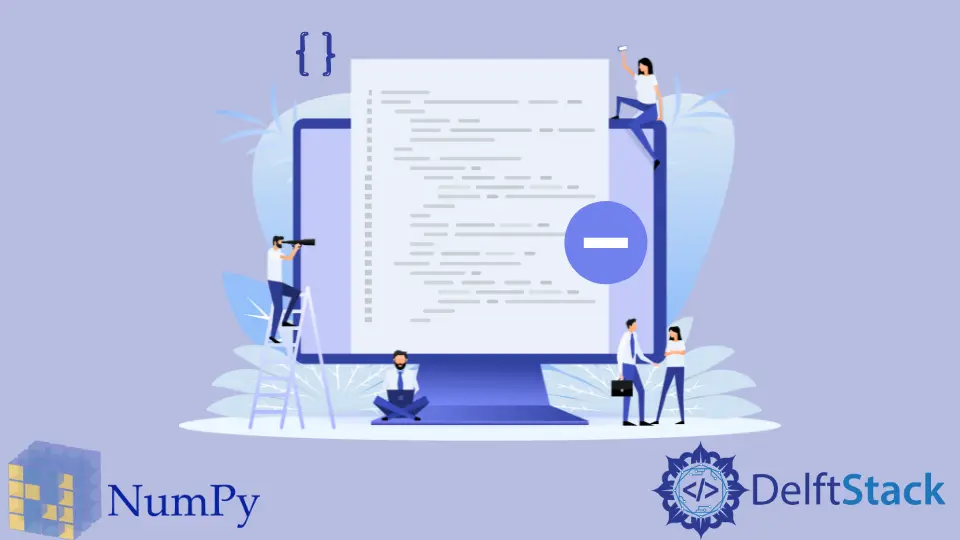
This tutorial will discuss the method to perform matrix subtraction operation in NumPy.
NumPy Matrix Subtraction With the -
Operator
The infix subtraction operator -
can be used to perform matrix subtraction in NumPy.
import numpy as np
matA = np.matrix([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
matB = np.matrix([[9, 8, 7], [6, 5, 4], [3, 2, 1]])
matC = matA - matB
print(matC)
Output:
[[-8 -6 -4]
[-2 0 2]
[ 4 6 8]]
We subtracted the matrix matB
from the matrix matB
with the -
operator in the above code. We first created the two matrices with the np.matrix()
function. We then performed matrix subtraction and saved the result inside the matrix matC
with matC = matA - matB
.
We can also perform the same subtraction using 2D arrays with the np.array()
instead of matrices. The following code example shows how to perform matrix subtraction using 2-dimensional arrays.
import numpy as np
matA = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
matB = np.array([[9, 8, 7], [6, 5, 4], [3, 2, 1]])
matC = matA - matB
print(matC)
Output:
[[-8 -6 -4]
[-2 0 2]
[ 4 6 8]]
The above code gives the same result as the previous example because there is no difference in the -
operator working with matrices and 2D arrays. It is because the np.matix
is a subclass of np.ndarray
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn