How to Calculate the Power of a NumPy Matrix
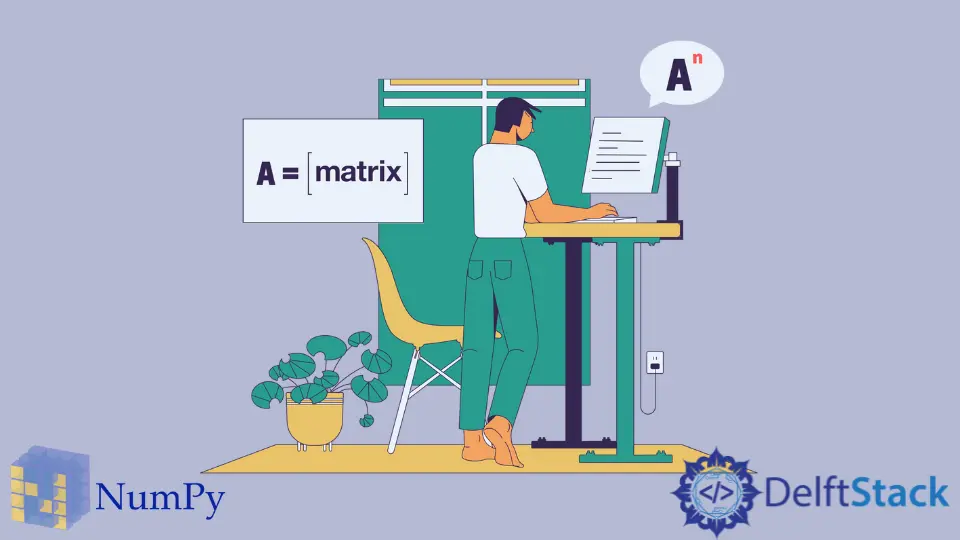
This article will introduce how to calculate the power of a matrix in NumPy.
Calculate the Power of a NumPy Matrix With the numpy.linalg.matrix_power()
Function
The matrix_power()
function inside the numpy.linalg
library is used to calculate the power of the matrix. It takes the matrix and the exponent as input parameters and returns the result of the operation in another matrix. See the following code example.
import numpy as np
matrix = np.array([[1, 2], [4, 5]])
power = np.linalg.matrix_power(matrix, 3)
print(power)
Output:
[[ 57 78]
[156 213]]
We first created the matrix as a 2D NumPy array with the np.array()
function in the above code. We then calculated the cube of the matrix with the matrix_power()
function and stored the result inside the power
matrix. In the end, we displayed the contents of the power
matrix. Keep in mind that this method only works with square matrices and gives an error if we try it on a rectangular matrix.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn