NumPy Matrix Indexing
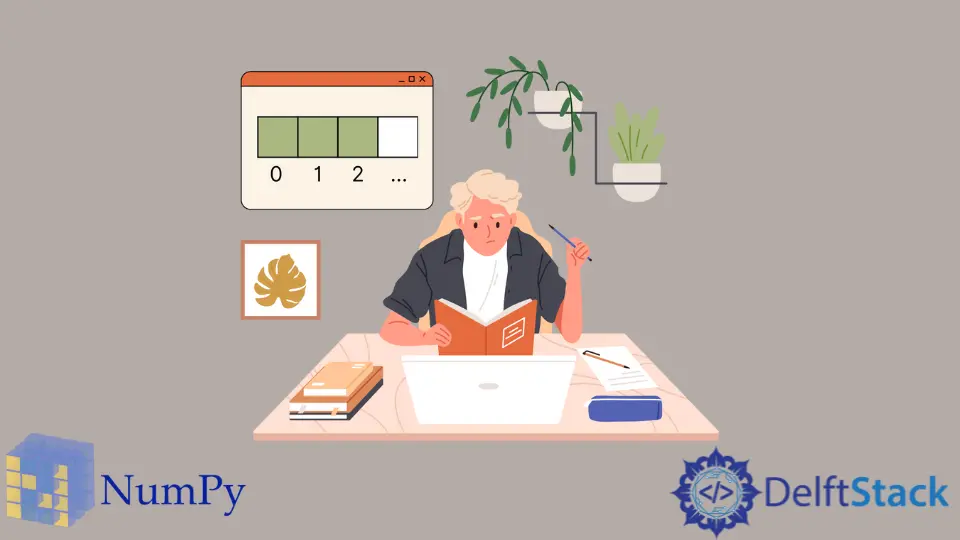
This tutorial will introduce the methods to specify the NumPy matrix index.
NumPy Matrix Indexing
Array indexing is used to access elements by specifying their indices inside the array. If we have an array filled with zeros and want to put a particular value at a specific index inside the array, we can use the array indexing method. Array indexing works very differently for 1D and 2D arrays in Python. If we want to access the first two elements of the 2D array as we did with the 1D array, we have to use Array[(0,1),(0,1)]
index.
import numpy as np
matrix = np.zeros((3, 3))
values = np.array([1, 2, 3])
matrix[(0, 1, 2), (0, 1, 2)] = values
print(matrix)
Output:
[[1. 0. 0.]
[0. 2. 0.]
[0. 0. 3.]]
We replaced the zeros at specific indices in our matrix matrix
with the values inside the values
array using the NumPy matrix indexing. We first created a matrix matrix
and filled it with zeros. We then created the array values
containing values that we want to input into our matrix. We then accessed the values inside the matrix with matrix[(0,1,2),(0,1,2)] = values
. It replaces the values at the indices 0,0
, 1,1
, and 2,2
of the matrix
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn