How to Map a Function in NumPy
-
Map a Function in NumPy With the
numpy.vectorize()
Function -
Map a Function in NumPy With the
lambda
Keyword in Python
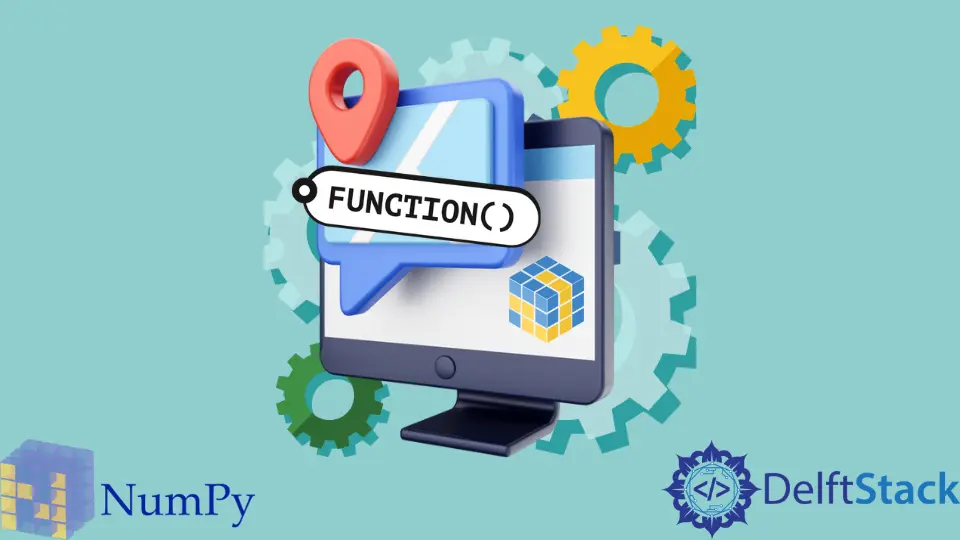
This tutorial will introduce the methods to map a function over a NumPy array in Python.
Map a Function in NumPy With the numpy.vectorize()
Function
The numpy.vectorize()
function maps functions on data structures that contain a sequence of objects like arrays in Python. It successively applies the input function on each element of the sequence or array. The return-type of the numpy.vectorize()
function is determined by the input function. See the following code example.
import numpy as np
array = np.array([1, 2, 3, 4, 5])
def fun(e):
return e % 2
vfunc = np.vectorize(fun)
result = vfunc(array)
print(result)
Output:
[1 0 1 0 1]
We first created the array
with the np.array()
function and declared the function fun
. Then we passed the fun
function to the np.vectorize()
function and stored the result in vfunc
. After that, we passed the array
to the vfunc
and stored the result inside the result
array.
Map a Function in NumPy With the lambda
Keyword in Python
The lambda
keyword creates an anonymous function in Python. Anonymous functions are helpful when we only need a function temporarily in our code. We can also use the lambda functions to map a function over a NumPy array. We can pass an array to the lambda function to apply iteratively over each array element.
import numpy as np
array = np.array([1, 2, 3, 4, 5])
def lfunc(e):
return e % 2
result = lfunc(array)
print(result)
Output:
[1 0 1 0 1]
We first created the array
with the np.array()
function and the lambda function lfunc
with the lambda
keyword. We then mapped the lfunc
to the array
by passing array
to the lfunc
function. We saved the result inside the result
array and printed the values inside it.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn