NumPy logspace() Function
- What is the NumPy logspace() Function?
- Using numpy.logspace() in Python
- Customizing the Output of logspace()
- Practical Applications of logspace()
- Conclusion
- FAQ
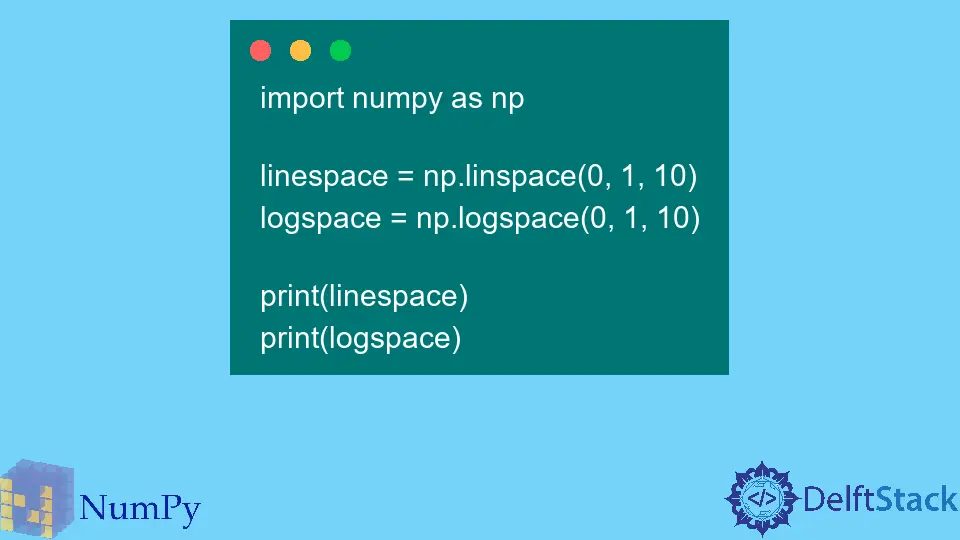
When working with numerical data in Python, the NumPy library is often a go-to resource for efficient computations. Among its many features, the numpy.logspace()
function stands out for its ability to generate logarithmically spaced values. This can be particularly useful in various scientific and engineering applications, where data often spans several orders of magnitude. Unlike numpy.linspace()
, which provides evenly spaced values, numpy.logspace()
focuses on the logarithmic scale, allowing for a more intuitive representation of data that grows exponentially.
In this article, we will explore how to effectively use the logspace()
function, complete with practical examples and clear explanations.
What is the NumPy logspace() Function?
The numpy.logspace()
function generates numbers that are spaced evenly on a logarithmic scale. This is especially helpful when you need to work with exponential data or when you want to visualize data that covers a large range. The function takes three main parameters: the starting exponent, the ending exponent, and the number of samples to generate. By specifying these parameters, you can create a range of values that are exponentially spaced, making it easier to analyze and visualize data.
For example, if you wanted to generate values between 10^1 and 10^3, you would use logspace()
to create an array of values that grow exponentially between these two points. This function is particularly useful in scientific computing, machine learning, and data analysis, where understanding the behavior of data across different scales is crucial.
Using numpy.logspace() in Python
To use the numpy.logspace()
function, you’ll first need to ensure that you have the NumPy library installed. If you haven’t installed it yet, you can do so using pip:
pip install numpy
Once you have NumPy ready, you can start using the logspace()
function. Here’s a simple example that demonstrates how to generate logarithmically spaced values:
import numpy as np
log_space_values = np.logspace(1, 3, num=5)
print(log_space_values)
Output:
[ 10. 31.6227766 100. 316.22776602 1000. ]
In this example, we imported the NumPy library and utilized the logspace()
function to generate five values between 10^1 (10) and 10^3 (1000). The parameters 1
and 3
represent the starting and ending exponents, respectively, while num=5
specifies that we want five values in total. The resulting output is an array of logarithmically spaced values that can be utilized in further calculations or visualizations.
Customizing the Output of logspace()
You can further customize the output of the logspace()
function by modifying its parameters. For instance, you can change the base of the logarithm or control whether the endpoint is included. By default, logspace()
uses a base of 10, but you can specify a different base using the base
parameter. Here’s how you can do that:
log_space_custom = np.logspace(0, 2, num=5, base=2)
print(log_space_custom)
Output:
[ 1. 2. 4. 8. 16. ]
In this example, we generated values between 2^0 (1) and 2^2 (4) using a base of 2. The output shows five logarithmically spaced values between the specified range. This flexibility in customizing the base allows you to adapt the logspace()
function to suit your specific needs, whether you’re working in binary, natural logarithm, or any other logarithmic scale.
Practical Applications of logspace()
The numpy.logspace()
function is not just a theoretical tool; it has practical applications in various fields. For example, in data visualization, logarithmic scales are often used to represent data that spans several orders of magnitude. This can be particularly useful in fields like finance, where stock prices can vary widely, or in scientific research, where measurements can differ by several orders of magnitude.
Here’s an example of how you might use logspace()
to create a dataset for plotting:
import matplotlib.pyplot as plt
x = np.logspace(0, 3, num=100)
y = np.sqrt(x)
plt.plot(x, y)
plt.xscale('log')
plt.title('Square Root Function on a Logarithmic Scale')
plt.xlabel('X values (Log Scale)')
plt.ylabel('Y values')
plt.grid(True)
plt.show()
In this example, we generated 100 logarithmically spaced values between 10^0 (1) and 10^3 (1000) for the x-axis. We then computed the square root of these values for the y-axis. By using plt.xscale('log')
, we ensured that the x-axis is displayed on a logarithmic scale, allowing for a clearer visualization of the relationship between the two variables. This approach is common in scientific papers and presentations, where clarity and precision are paramount.
Conclusion
The numpy.logspace()
function is an invaluable tool for generating logarithmically spaced values in Python. Its ability to create values across a wide range makes it ideal for various applications, from scientific research to data visualization. By understanding how to customize its parameters, you can tailor the output to meet your specific needs, enhancing your data analysis capabilities. Whether you’re a seasoned data scientist or just starting out, mastering the logspace()
function can significantly improve your workflow and the clarity of your data presentations.
FAQ
-
what is the difference between numpy.linspace() and numpy.logspace()?
numpy.linspace() generates evenly spaced values, while numpy.logspace() generates values spaced evenly on a logarithmic scale. -
can I change the base of the logarithm in numpy.logspace()?
yes, you can specify a different base using the base parameter in thelogspace()
function. -
how can I visualize data generated by numpy.logspace()?
you can use libraries like matplotlib to plot data generated by logspace(), allowing for clear visualization on a logarithmic scale. -
is numpy.logspace() only useful for scientific applications?
while it is commonly used in scientific applications, numpy.logspace() can also be useful in finance, machine learning, and any field that requires analysis of data across different scales. -
can I include the endpoint in the output of numpy.logspace()?
yes, by default, numpy.logspace() includes the endpoint, but you can adjust the parameters to control the output as needed.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn