NumPy Factorial
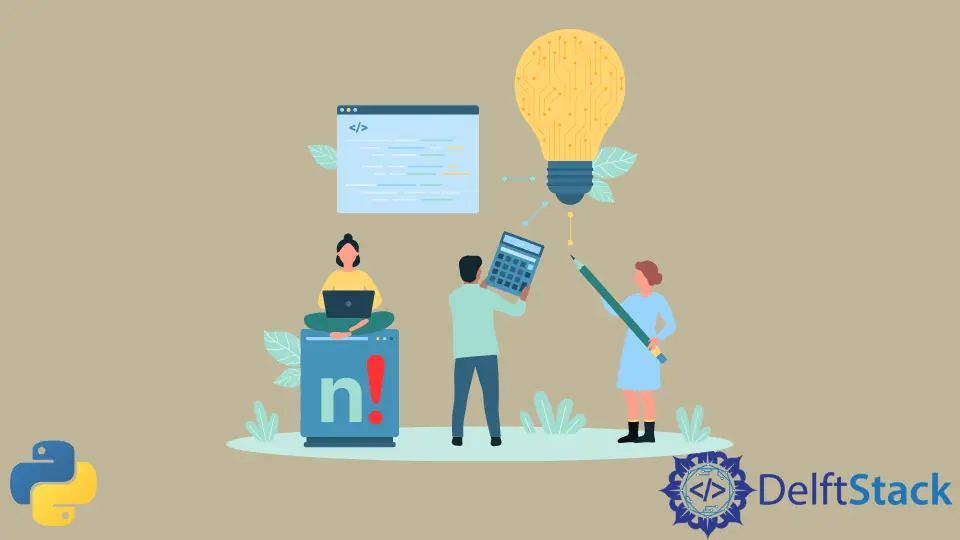
This tutorial will introduce the method to calculate the element-wise factorial of a NumPy array in Python.
NumPy Factorial With the factorial()
Function From Scipy
Suppose we have an array that consists of numerical values and want to calculate the factorial of each element of the array. In that case, we can use the factorial()
function inside the scipy
package of Python. The scipy
package is an external package and does not come pre-installed with the Python programming language. The command to install the scipy
package is given below.
pip install scipy
The factorial()
function takes the array as an argument, carries out element-wise factorial, and returns an array that contains the calculated factorials.
from scipy.special import factorial
import numpy as np
array = np.array([[1, 3, 5], [2, 4, 6]])
factorials = factorial(array)
print(factorials)
Output:
[[ 1. 6. 120.]
[ 2. 24. 720.]]
In the above code, we calculated the element-wise factorial of the NumPy array array
with the factorial()
function inside the scipy.special
package. We first created the NumPy array array
with the np.array()
function. We then calculated the element-wise factorial with the factorial()
function and stored the output inside another NumPy array, factorials
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn