NumPy Array Equal
-
NumPy Arrays Equality Check With the
==
Operator in Python -
NumPy Arrays Equality Check With the
numpy.array_equal()
Function -
NumPy Arrays Equality Check With the
numpy.array_equiv()
Function in Python -
NumPy Equal With the
numpy.allcloses()
Function in Python
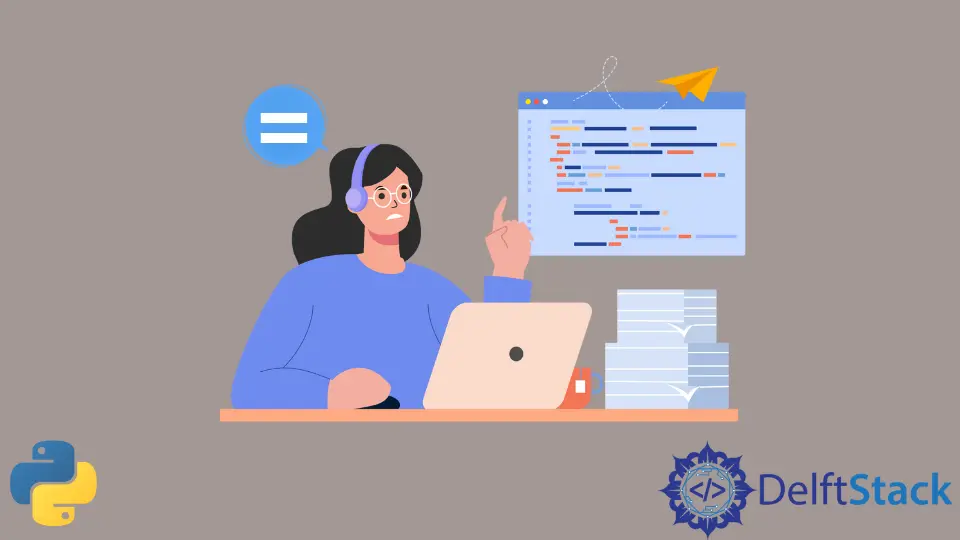
This article will introduce the methods to carry out element-wise equality comparison on NumPy arrays in Python.
NumPy Arrays Equality Check With the ==
Operator in Python
The ==
equality comparison operator is used to check whether two quantities are equal or not. The ==
operator returns True
if the quantities are equal and False
if the quantities are not equal. We can use the ==
operator along with the all()
function to check whether all the elements of the two arrays are equal or not. The following code example shows us how we can element-wise compare two arrays for equality with the ==
operator in Python.
import numpy as np
array1 = np.array([1, 2, 3, 4, 5])
array2 = np.array([1, 2, 3, 4, 5])
print((array1 == array2).all())
Output:
True
In the above code, we element-wise compared the arrays array1
and array2
for equality with the ==
operator and the all()
function. We first created the arrays array1
and array2
with the np.array()
function. We then used the ==
operator with the all()
function to check if all the values inside array1
are equal to the values inside array2
. This approach is very efficient and easy to understand, but there are a few disadvantages to using this approach. For example, if either of the arrays is empty and the second array contains only one element, this approach will return a True
value. Another problem is that if both arrays have different shapes, this approach will give us an error.
NumPy Arrays Equality Check With the numpy.array_equal()
Function
A more thorough and error-free way of achieving the same objective as the previous approach is to use the numpy.array_equal()
function. The numpy.array_equal()
function compares two arrays for equality. The numpy.array_equal()
function returns True
if the arrays are equal and False
if the arrays are not equal. The following code example shows us how we can element-wise compare two arrays for equality with the numpy.array_equal()
function.
import numpy as np
array1 = np.array([1, 2, 3, 4, 5])
array2 = np.array([1, 2, 3, 4, 5])
print(np.array_equal(array1, array2))
Output:
True
In the above code, we used the np.array_equal()
function to check if all the values inside array1
are equal to the values inside array2
.
NumPy Arrays Equality Check With the numpy.array_equiv()
Function in Python
The numpy.array_equiv()
function can also be used to check whether two arrays are equal or not in Python. The numpy.array_equiv()
function returns True
if both arrays have the same shape and all the elements are equal, and returns False
otherwise.
import numpy as np
array1 = np.array([1, 2, 3, 4, 5])
array2 = np.array([0, 2, 3, 4, 5])
print(np.array_equiv(array1, array2))
Output:
False
In the above code, we used the np.array_equiv()
function to check if array1
is equal to array2
.
NumPy Equal With the numpy.allcloses()
Function in Python
The numpy.allclose()
function can also be used to check if two arrays are element-wise equal or not in Python. The numpy.allclose()
function returns True
if all the elements inside both arrays are equal within a specified tolerance.
import numpy as np
array1 = np.array([1, 2, 3, 4, 5])
array2 = np.array([1, 2, 3, 4, 5])
print(np.allclose(array1, array2))
Output:
False
In the above code, we used the np.allclose()
function to check if array1
is equal to array2
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn