How to Delete Row in NumPy
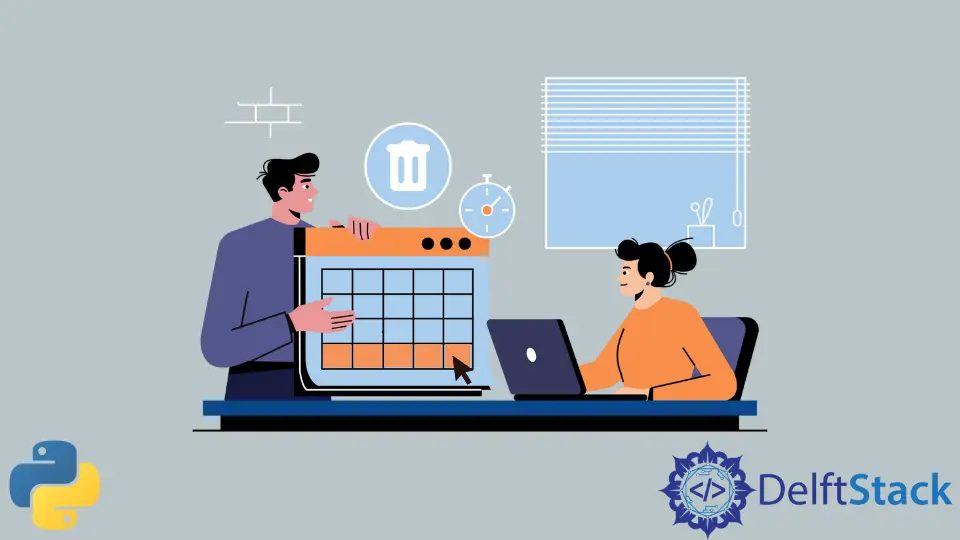
This tutorial will introduce how to delete a row from a multi-dimensional NumPy array in Python.
Delete NumPy Row With the numpy.delete()
Function
If we have a multi-dimensional NumPy array and want to delete a particular row from it, we can use the numpy.delete()
function. The numpy.delete()
function deletes entries at a specific index along a specified axis from a NumPy array. The numpy.delete()
function takes the array, the index to be deleted, and the axis along which we want to delete as arguments and returns a sub-array in which the specified index along with the specified axis is deleted. The following code example shows us how we can delete a row from a multi-dimensional array with the numpy.delete()
function.
import numpy as np
array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
array = np.delete(array, (1), axis=0)
print(array)
Output:
[[1 2 3]
[7 8 9]]
We deleted the second row from the 2-dimensional NumPy array array
with the np.delete()
function in the above code. We first created the 2-dimensional NumPy array array
with the np.array()
function. Then we deleted the entries at index 1
along the axis 0
, which is the second row of the array
, and saved the result in array
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn