NumPy Deep Copy
-
NumPy Deep Copy With the
copy.deepcopy()
Function in Python - NumPy Deep Copy With the User-Defined Approach in Python
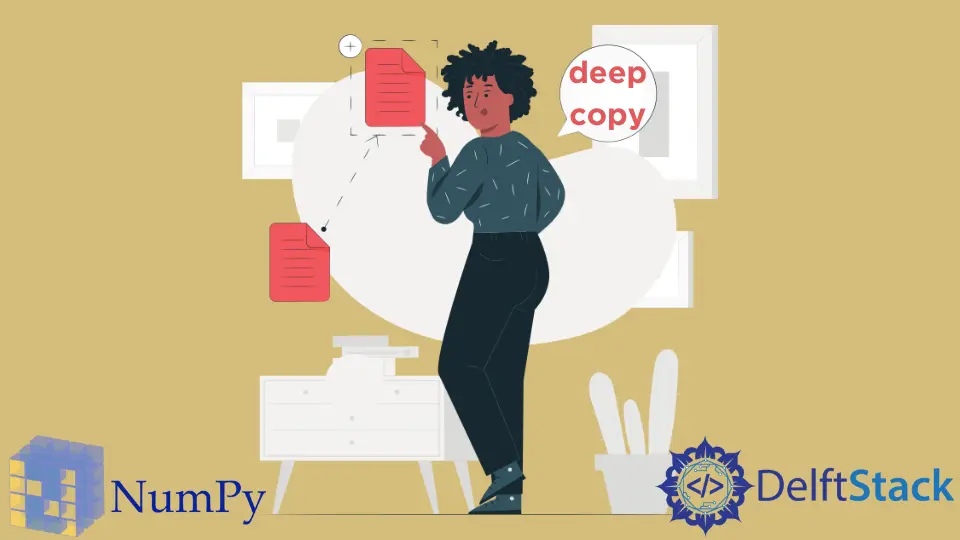
This tutorial will introduce the methods to deep copy a NumPy array in Python.
NumPy Deep Copy With the copy.deepcopy()
Function in Python
Python has two types of copies, a shallow copy and a deep copy. A shallow copy means the copied array contains only a reference to the original array. It means that any change in the original array will be reflected inside the copied array. On the other hand, a deep copy means copying each element of the original array into the copied array. In this type of copying, a new memory location is allocated to each element inside the copied array. This means that any change in the original array will not change anything inside the copied array.
The deepcopy()
function inside the copy
module is used to deep copy lists, but it also works just fine with arrays in Python. The copy.deepcopy()
function takes the array as an input argument and returns a deep copy of the array. The following code example shows us how to deep copy a NumPy array with the copy.deepcopy()
function in Python.
import numpy as np
import copy
array = np.array([1, 2, 3, 4])
array2 = copy.deepcopy(array)
array[0] = array[0] + 1
print(array)
print(array2)
Output:
[2 2 3 4]
[1 2 3 4]
In the above code, we deep copied the NumPy array array
inside the array2
with the copy.deepcopy()
function. We then modified the elements inside the array
. The output shows that changing the values inside the NumPy array array
has no effect on the NumPy array array2
.
NumPy Deep Copy With the User-Defined Approach in Python
Another method of deep copying a NumPy array is to iterate through the whole array and copy each element inside it. See the following code example.
import numpy as np
array = np.array([1, 2, 3, 4])
array2 = np.array([x for x in array])
array[1] = 1
print(array)
print(array2)
Output:
[1 1 3 4]
[1 2 3 4]
In the above code, we deep copied the NumPy array array
inside the NumPy array array2
by iterating through each element inside the array
. We then modified the elements inside the array
. The output shows that changing the values inside the NumPy array array
has no effect on the NumPy array array2
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn